1.09 Unit Test Narrative Techniques And Structure Part 1
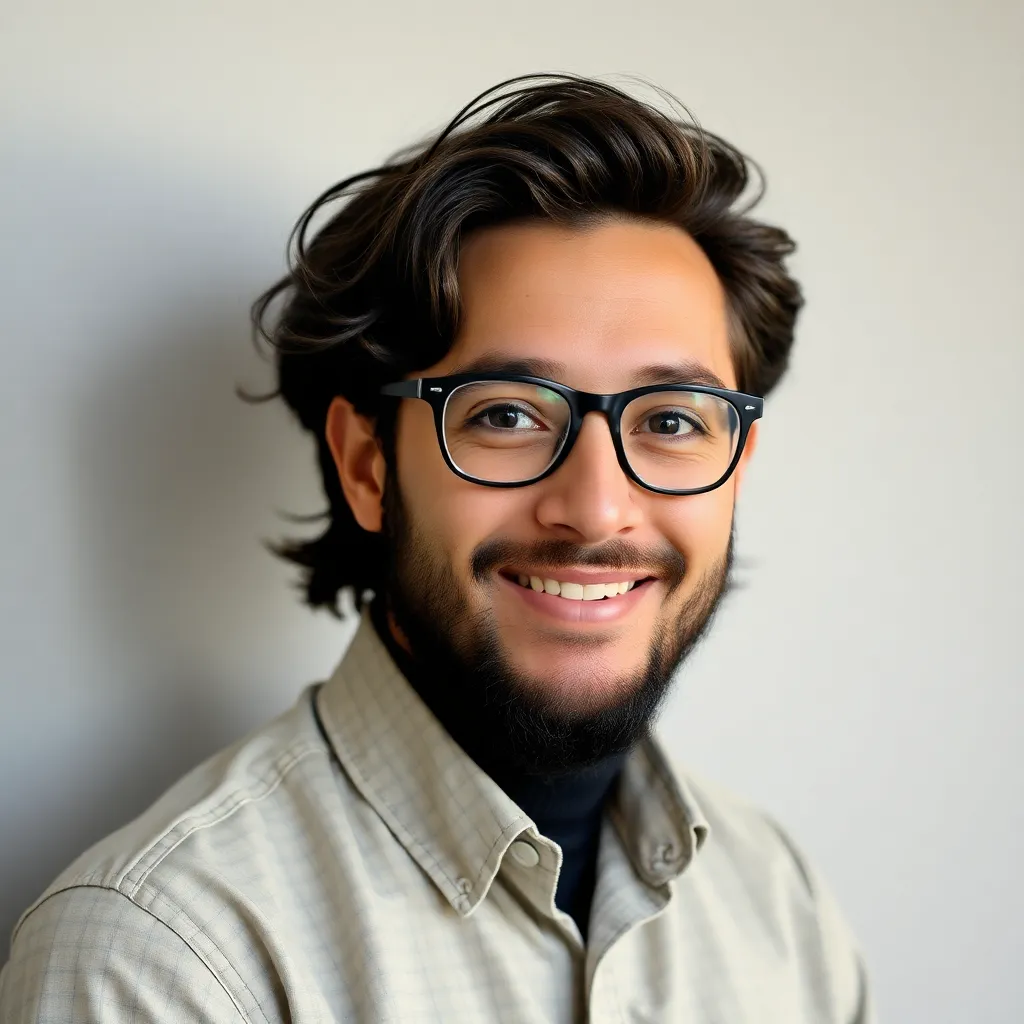
Breaking News Today
May 11, 2025 · 5 min read
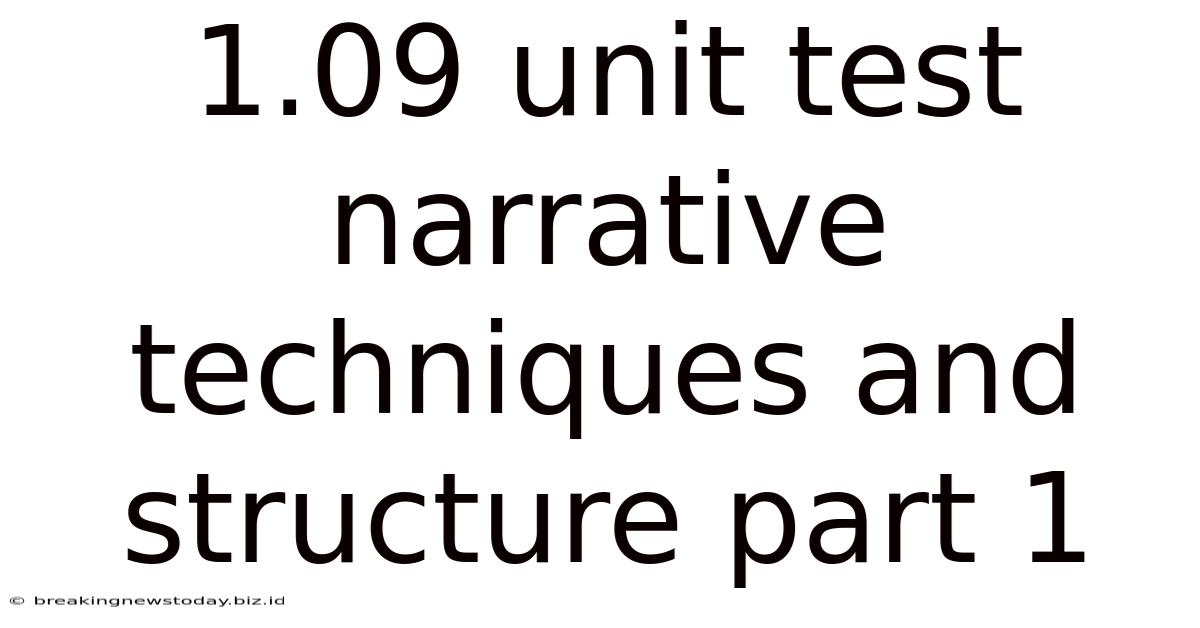
Table of Contents
1.09 Unit Test Narrative Techniques and Structure: Part 1 - Crafting Compelling and Effective Tests
Unit testing is the bedrock of robust software development. It's the process of verifying individual units or components of code work as expected in isolation. But writing effective unit tests isn't just about ensuring functionality; it's about crafting clear, concise, and maintainable narratives that communicate the why behind the test, as well as the what. This first part explores narrative techniques and structural best practices for creating 1.09 unit tests (assuming a context where "1.09" denotes a specific version or style guide, perhaps within a company or project).
The Importance of Narrative in Unit Tests
Many developers treat unit tests as mere functional checks. They focus solely on the assert
statements, neglecting the crucial role of descriptive names and insightful comments. However, a well-written unit test is more than a collection of assertions; it's a story. This story explains:
- The Context: What scenario is being tested? What are the preconditions?
- The Action: What is the unit of code doing? What inputs are provided?
- The Expected Outcome: What is the anticipated result? Why is this result expected?
- The Verification: How is the outcome verified? Are the assertions clear and unambiguous?
A strong narrative allows other developers (and your future self) to quickly understand the test's purpose and functionality without diving deep into the code. This drastically improves maintainability and reduces debugging time.
Structural Best Practices for 1.09 Unit Tests
The structure of your unit tests significantly influences readability and maintainability. Here are some key principles for 1.09 unit tests:
1. Descriptive Test Names: The Headline of Your Story
Test names are the first and often the only thing other developers see. Therefore, they must be informative and self-explanatory. Avoid generic names like test_function1()
or test_case_a()
. Instead, opt for names that clearly describe the scenario being tested:
- Bad:
test_calculate_total()
- Good:
test_calculate_total_with_zero_items_returns_zero()
- Better:
test_ShoppingCart_calculateTotal_withZeroItems_returnsZero()
(using class/method names for context)
The Given-When-Then pattern is a powerful technique for creating descriptive names:
- Given the shopping cart is empty
- When the
calculateTotal()
method is called - Then the total should be zero
This pattern translates directly into a readable test name: test_ShoppingCart_calculateTotal_GivenEmptyCart_ThenReturnsZero()
.
2. Arrange-Act-Assert (AAA) Pattern: The Structure of Your Story
The AAA pattern is a widely accepted best practice for organizing the logic within a unit test. It divides the test into three distinct phases:
- Arrange: Set up the necessary preconditions. This includes creating objects, initializing variables, and mocking dependencies.
- Act: Execute the unit of code under test. This is typically a single method call.
- Assert: Verify the outcome by comparing the actual result to the expected result. This uses assertions provided by your testing framework (e.g.,
assertEqual
,assertTrue
,assertRaises
).
Example (Python):
import unittest
class ShoppingCartTest(unittest.TestCase):
def test_ShoppingCart_calculateTotal_GivenEmptyCart_ThenReturnsZero(self):
# Arrange
cart = ShoppingCart() # Create an instance of the ShoppingCart class
# Act
total = cart.calculateTotal() # Call the method being tested
# Assert
self.assertEqual(total, 0) # Verify the result
This structure makes the test's flow easy to follow, improving readability and making it simpler to debug.
3. Keep Tests Focused and Independent: Short Chapters in Your Story
Each unit test should focus on a single aspect of the unit's functionality. Avoid creating tests that cover multiple scenarios within a single test function. This makes it harder to identify the source of failure when a test fails.
Similarly, ensure that tests are independent. One test should not depend on the state or outcome of another test. This is particularly important when running tests in parallel.
4. Meaningful Comments: Explaining the Narrative
While descriptive names and the AAA structure go a long way, comments can add further clarity, especially for complex scenarios or edge cases. Comments should explain why a particular approach or assertion is used, not what the code does (the code should be self-explanatory).
Example:
# Assert that the exception message contains the expected error details.
# This is crucial for ensuring the correct error handling is implemented.
self.assertIn("Invalid item ID", str(exception))
5. Consistent Style and Formatting: Maintaining a Coherent Narrative
Consistency is key to readability. Use consistent indentation, naming conventions, and commenting styles throughout your test suite. Adhere to your project's coding style guide or adopt a widely accepted style guide like PEP 8 (for Python).
Advanced Narrative Techniques for 1.09 Unit Tests
1. Using Mock Objects: Controlling the Narrative Environment
Mock objects are invaluable for isolating the unit under test from its dependencies. They allow you to simulate the behavior of external systems or components, providing predictable inputs and outputs. This simplifies testing and avoids the complexities of integrating with real-world systems.
For instance, if your unit interacts with a database, mocking the database interaction allows you to test the unit's logic without needing a database connection.
2. Parameterized Tests: Telling Multiple Variations of the Same Story
Parameterized tests allow you to run the same test with different inputs. This significantly reduces code duplication and improves test coverage. Many testing frameworks provide support for parameterized tests.
3. Property-Based Testing: Exploring a Wide Range of Narratives
Property-based testing is a powerful technique for automatically generating a large number of test cases based on specified properties or constraints. It can help uncover unexpected edge cases and improve test coverage.
4. Test-Driven Development (TDD): Shaping the Narrative from the Start
TDD is a development methodology where you write the unit tests before writing the code. This ensures that the code is written to meet specific requirements and improves the overall design. Writing the test first forces you to think carefully about the expected behavior and edge cases.
Conclusion: The Power of a Well-Told Story
Writing effective unit tests is more than simply verifying functionality; it's about crafting clear, concise, and maintainable narratives. By adopting structural best practices like the AAA pattern, using descriptive names and comments, and employing advanced techniques such as mocking and parameterized tests, you can create a unit test suite that is not only effective in ensuring code quality but also serves as a valuable documentation tool. Remember, a well-told story in your unit tests translates to easier maintenance, faster debugging, and a more robust and reliable software application. This first part has laid the foundation; the second part will delve deeper into specific examples and advanced scenarios within the 1.09 unit test framework.
Latest Posts
Latest Posts
-
A Partial Bath Includes Washing A Residents
May 12, 2025
-
Which Of The Following Describes A Net Lease
May 12, 2025
-
Nurse Logic 2 0 Knowledge And Clinical Judgment
May 12, 2025
-
Panic Disorder Is Characterized By All Of The Following Except
May 12, 2025
-
Positive Individual Traits Can Be Taught A True B False
May 12, 2025
Related Post
Thank you for visiting our website which covers about 1.09 Unit Test Narrative Techniques And Structure Part 1 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.