5.08 Unit Test Informational Works Part 1
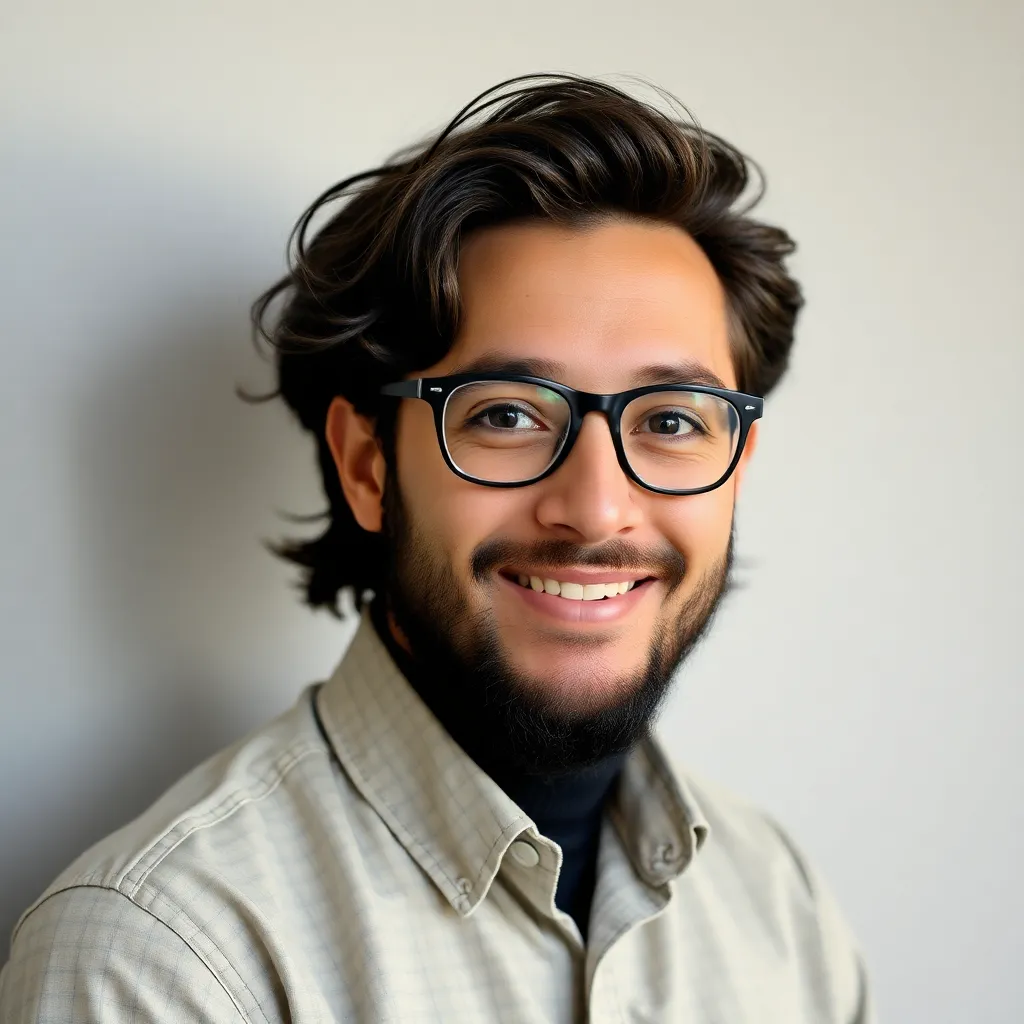
Breaking News Today
May 11, 2025 · 6 min read
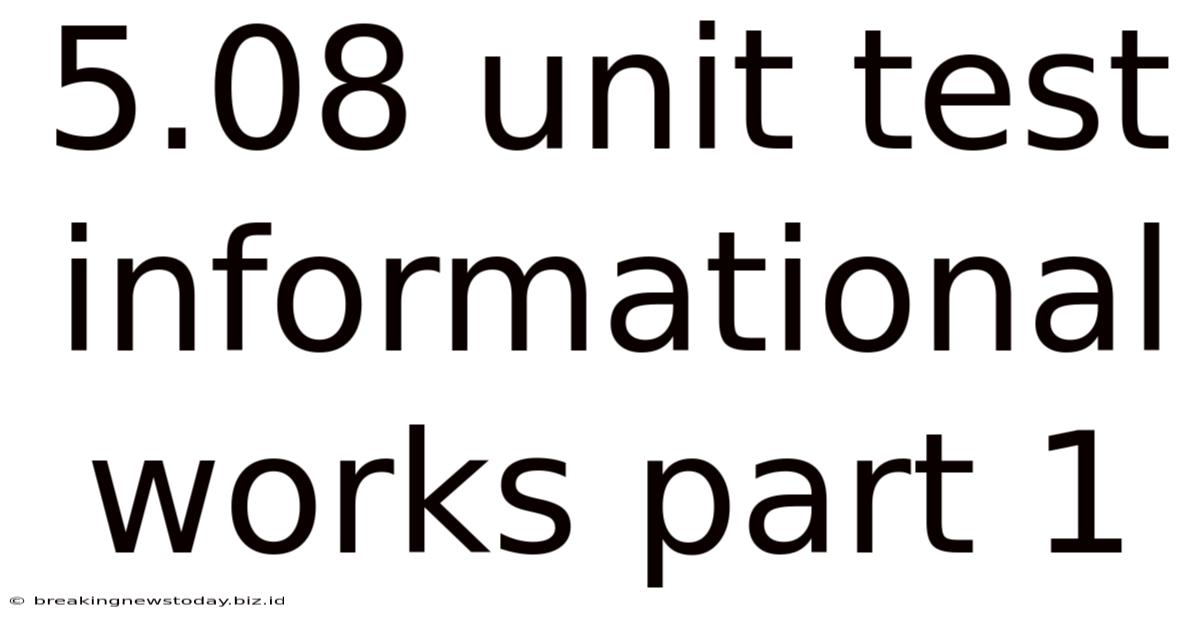
Table of Contents
5.08 Unit Test Informational Works: Part 1 - Laying the Foundation for Robust Software
Unit testing is a cornerstone of software development best practices. It involves testing individual components (units) of code in isolation to ensure they function correctly. This first part of a series on 5.08 unit testing will delve deep into its importance, methodologies, and essential concepts, laying the groundwork for creating robust and reliable software. We'll explore the "why," the "what," and the "how" of effective unit testing.
Why is Unit Testing Crucial?
In the fast-paced world of software development, delivering high-quality, bug-free applications is paramount. Unit testing significantly contributes to this goal by offering several critical benefits:
1. Early Bug Detection:
Catching bugs early in the development lifecycle is significantly cheaper and easier than fixing them later. Unit tests identify issues at the unit level, before they propagate to integration and system-level testing, saving considerable time and resources. Early detection prevents snowballing errors that can be incredibly complex and costly to resolve.
2. Improved Code Design:
Writing unit tests forces developers to think about code design from a testability perspective. This promotes the creation of modular, loosely coupled code, which is easier to maintain, understand, and extend. Testable code inherently tends to be better-designed code.
3. Enhanced Code Maintainability:
As software evolves, maintaining its integrity becomes challenging. Unit tests act as a safety net, allowing developers to refactor and modify code with confidence. If a change breaks something, the tests will immediately alert the developers, minimizing the risk of introducing regressions. A solid suite of unit tests acts as living documentation for the codebase.
4. Increased Developer Productivity:
While initially requiring extra effort, unit testing ultimately boosts developer productivity. By catching bugs early and preventing regressions, it reduces debugging time and frustration, allowing developers to focus on building new features and functionality. The upfront investment in testing pays dividends in the long run.
5. Reduced Risk:
Thorough unit testing minimizes the risk of deploying faulty software. By identifying and addressing issues before release, it increases customer satisfaction and reduces the likelihood of costly recalls or patches. Confidence in the software's reliability is invaluable.
What is a Unit Test?
A unit test focuses on verifying the behavior of a single, isolated unit of code. This unit can be a function, method, module, or a small class. The goal is to test the unit's functionality independently of other parts of the system. This isolation is key to achieving accurate and reliable test results.
Key Characteristics of a Good Unit Test:
- Independent: The test should not depend on external factors or other units. Mocking or stubbing dependencies is often necessary to achieve this independence.
- Repeatable: The test should produce the same results every time it's run, regardless of the environment.
- Fast: Unit tests should execute quickly to allow for frequent execution during development. Slow tests discourage their regular use.
- Self-Validating: The test should automatically determine whether it passed or failed, without manual intervention.
- Specific: Each test should focus on a specific aspect of the unit's functionality. Avoid testing multiple things in a single test.
How to Write Effective Unit Tests:
Writing effective unit tests requires careful planning and a systematic approach. Here are some essential steps:
1. Choose a Testing Framework:
Numerous testing frameworks are available for various programming languages. Some popular examples include:
- Python:
unittest
,pytest
- Java: JUnit, TestNG
- JavaScript: Jest, Mocha, Jasmine
- C#: NUnit, MSTest, xUnit
Selecting the right framework depends on your language and project requirements. Many frameworks offer features like test runners, assertion libraries, and mocking capabilities.
2. Define Test Cases:
Before writing any code, carefully identify the different scenarios and edge cases your unit should handle. Each scenario should be translated into a separate test case. Consider both positive (expected behavior) and negative (error handling) scenarios. Thorough test case design is crucial for comprehensive testing.
3. Write Clear and Concise Tests:
Each test should be clear, concise, and focused on a single aspect of the unit's behavior. Use descriptive test names that clearly indicate what is being tested. Leverage the framework's assertion methods to verify the expected outcomes. Good naming conventions like test_<function_name>_<scenario>
greatly enhance readability and maintainability.
4. Use Mocking and Stubbing:
When testing a unit that interacts with external dependencies (databases, APIs, file systems), mocking or stubbing these dependencies is crucial. Mocking simulates the behavior of a dependency without actually interacting with it. Stubbing provides predefined responses to the unit's requests, allowing you to focus solely on the unit's internal logic. This isolates the unit under test, preventing external factors from affecting the test results.
5. Run Tests Frequently:
Integrate unit testing into your development workflow. Run tests frequently, ideally after every code change, to detect bugs early. Many IDEs and build tools offer seamless integration with testing frameworks, simplifying the testing process. Continuous integration (CI) systems can further automate the testing process.
6. Aim for High Test Coverage:
While 100% test coverage is a challenging goal, striving for high coverage is beneficial. Test coverage metrics provide insights into the extent to which your code is tested. High coverage helps identify untested areas and improve the overall robustness of your software. Remember that high coverage alone isn't sufficient; the tests themselves must be well-designed and effective.
Example: A Simple Python Unit Test using unittest
Let's illustrate these concepts with a simple Python example:
import unittest
def add(x, y):
"""Adds two numbers."""
return x + y
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-2, -3), -5)
def test_add_zero(self):
self.assertEqual(add(5, 0), 5)
def test_add_mixed_numbers(self):
self.assertEqual(add(-2, 5), 3)
if __name__ == '__main__':
unittest.main()
This example demonstrates the basic structure of a unit test using Python's unittest
framework. Each test_
method represents a separate test case, verifying different aspects of the add
function's behavior. The assertEqual
method asserts that the actual result matches the expected result.
Beyond the Basics: Advanced Unit Testing Techniques
This initial exploration provides a foundational understanding of unit testing. Future parts in this series will delve into more advanced techniques, including:
- Test-Driven Development (TDD): Writing tests before writing the code.
- Mock Object Frameworks: Advanced mocking techniques for complex dependencies.
- Integration Testing: Testing the interaction between multiple units.
- Code Coverage Analysis: Measuring the effectiveness of your test suite.
- Continuous Integration/Continuous Delivery (CI/CD): Automating the testing and deployment process.
Mastering these advanced techniques will empower you to create truly robust and reliable software. By embracing a culture of thorough unit testing, you significantly reduce the risk of defects and improve the overall quality of your software projects. Remember, investing time in effective unit testing is an investment in the long-term success of your software.
Latest Posts
Latest Posts
-
The Proper Technique For Using The Power Grip Is To
May 11, 2025
-
Move To The Safety Shower If You Spill
May 11, 2025
-
Some Businesses Avoid Risks By Doing Which Of The Following
May 11, 2025
-
The Correct Reference Book For Hospital Procedures Is
May 11, 2025
-
What Do The Icons In This Image Represent
May 11, 2025
Related Post
Thank you for visiting our website which covers about 5.08 Unit Test Informational Works Part 1 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.