Create A Single Record Form From The Classes Table
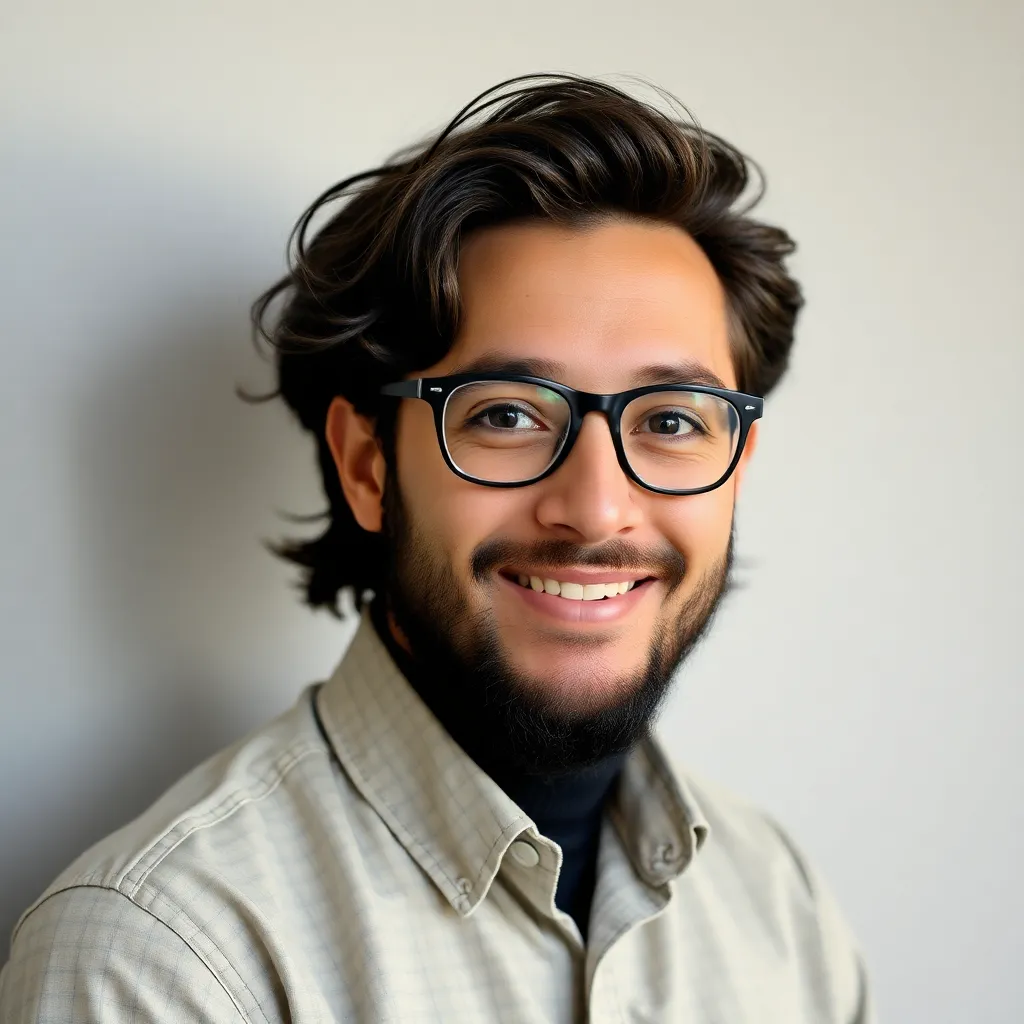
Breaking News Today
Apr 01, 2025 · 6 min read
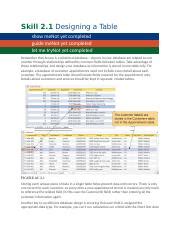
Table of Contents
Create a Single Record Form from the Classes Table: A Comprehensive Guide
Creating a single record form from a database table is a fundamental task in web development. This comprehensive guide will walk you through the process of building a form to manage individual records from a "Classes" table, covering various aspects from database design to front-end implementation and back-end processing. We'll focus on clarity and best practices, ensuring your form is both user-friendly and efficient.
Understanding the Database Structure
Before diving into the form creation, let's define our "Classes" table. A typical "Classes" table might contain columns like:
- classID (INT, PRIMARY KEY): Unique identifier for each class. This is crucial for identifying and updating specific records.
- className (VARCHAR): The name of the class (e.g., "Introduction to Programming," "Advanced Calculus").
- instructorID (INT, FOREIGN KEY): Links to an "Instructors" table (not covered in this example, but essential for a real-world application).
- description (TEXT): A detailed description of the class.
- credits (INT): Number of credits awarded for completing the class.
- startDate (DATE): The start date of the class.
- endDate (DATE): The end date of the class.
Designing the User Interface (UI)
The UI should be intuitive and easy to use. We'll use HTML for the structure and CSS for styling. Consider these elements:
- Clear Labels: Each input field should have a clear label explaining what information to enter.
- Appropriate Input Types: Use the correct HTML input type for each field (e.g.,
<input type="text">
for text fields,<input type="date">
for dates,<textarea>
for longer descriptions). - Input Validation: Implement client-side validation (using JavaScript) to prevent invalid data from being submitted. This improves user experience by providing immediate feedback.
- Error Handling: Display clear error messages if the validation fails.
- User-Friendly Layout: Organize the form elements logically, perhaps grouping related fields together. Consider using a responsive design to ensure the form looks good on different screen sizes.
Here's an example of a basic HTML form structure:
Remember to replace "submit_class.php"
with the actual name of your server-side script. The required
attribute ensures that users fill out all necessary fields. The readonly
attribute on classID
is important if you are updating an existing record, preventing accidental changes to the primary key.
Adding Client-Side Validation with JavaScript
Client-side validation enhances the user experience by providing immediate feedback. Here's a simple example using JavaScript to check if the class name is filled:
document.getElementById("classForm").addEventListener("submit", function(event) {
if (document.getElementById("className").value === "") {
alert("Please enter a class name.");
event.preventDefault(); // Prevent form submission
}
});
This script checks if the "className" field is empty. You would expand this to include validation for all required fields, data types, and other constraints. Consider using a JavaScript library like jQuery to simplify the process.
Server-Side Processing (PHP Example)
The server-side script handles the form submission, connects to the database, and performs the necessary operations (insertion or update). Here's an example using PHP and MySQLi:
connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Check if the form was submitted
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// Get form data
$classID = $_POST["classID"];
$className = $_POST["className"];
// ... get other form data ...
// Prepare and bind SQL statement (prevent SQL injection!)
if (empty($classID)){ //insert new record
$sql = "INSERT INTO Classes (className, instructorID, description, credits, startDate, endDate) VALUES (?, ?, ?, ?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("siisss", $className, $instructorID, $description, $credits, $startDate, $endDate);
} else { //update existing record
$sql = "UPDATE Classes SET className = ?, instructorID = ?, description = ?, credits = ?, startDate = ?, endDate = ? WHERE classID = ?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("siisss", $className, $instructorID, $description, $credits, $startDate, $endDate, $classID);
}
// Execute the query
if ($stmt->execute()) {
echo "Record updated successfully";
} else {
echo "Error updating record: " . $stmt->error;
}
$stmt->close();
}
$conn->close();
?>
This code snippet demonstrates both insertion (when classID
is empty) and updating (when classID
is provided) of records. Crucially, it uses prepared statements to prevent SQL injection vulnerabilities. This is vital for security. Remember to replace the placeholder database credentials with your actual details.
Error Handling and User Feedback
Robust error handling is essential. The PHP code includes basic error checking, but you should expand this to handle more specific errors (e.g., duplicate class names, invalid data types). Provide clear and informative messages to the user, indicating what went wrong and how to correct the issue.
Improving the User Experience
Consider these enhancements to improve the user experience:
- Autocompletion: For fields like
instructorID
, implement autocompletion to suggest instructors based on their ID or name. This reduces typing and errors. - Date Pickers: Instead of relying solely on the HTML5
<input type="date">
, consider using a JavaScript date picker library for better user interaction. - Progress Indicators: For large forms or operations involving database interaction, display a progress indicator to keep the user informed.
- Confirmation Dialogs: Before submitting changes to an existing record, display a confirmation dialog to prevent accidental data loss.
Security Considerations
Security should be a top priority. Always use prepared statements or parameterized queries to prevent SQL injection attacks. Sanitize all user inputs before using them in your queries. Consider using a framework or library that handles these security aspects for you. Also, protect your database credentials carefully and avoid exposing them in your code.
Testing and Deployment
Thoroughly test your form to ensure it works correctly under various conditions. Test both insertion and updating functionality. Check error handling and validation. Use a testing framework if possible. Once thoroughly tested, deploy your form to your web server. Regularly update your code and database schema to address any bugs or security vulnerabilities.
Conclusion
Creating a single record form for your "Classes" table involves careful planning and implementation. By following these steps, combining effective UI design with robust back-end processing and comprehensive error handling, you can create a functional, user-friendly, and secure form to manage your class data efficiently. Remember to prioritize security best practices to protect your database and your users. Continuous testing and improvement will ensure your form remains reliable and effective.
Latest Posts
Latest Posts
-
Bach Created Masterpieces In Every Baroque Genre Except
Apr 03, 2025
-
A Defining Characteristic Of American Politics Is
Apr 03, 2025
-
Hardware Lab Simulation 7 1 Investigating Network Connection Settings
Apr 03, 2025
-
In Cell B9 Enter A Formula Using Npv
Apr 03, 2025
-
All Of The Following Are Roles Of Protein Except
Apr 03, 2025
Related Post
Thank you for visiting our website which covers about Create A Single Record Form From The Classes Table . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.