Create An Automatic Basic Report From The Classes Table
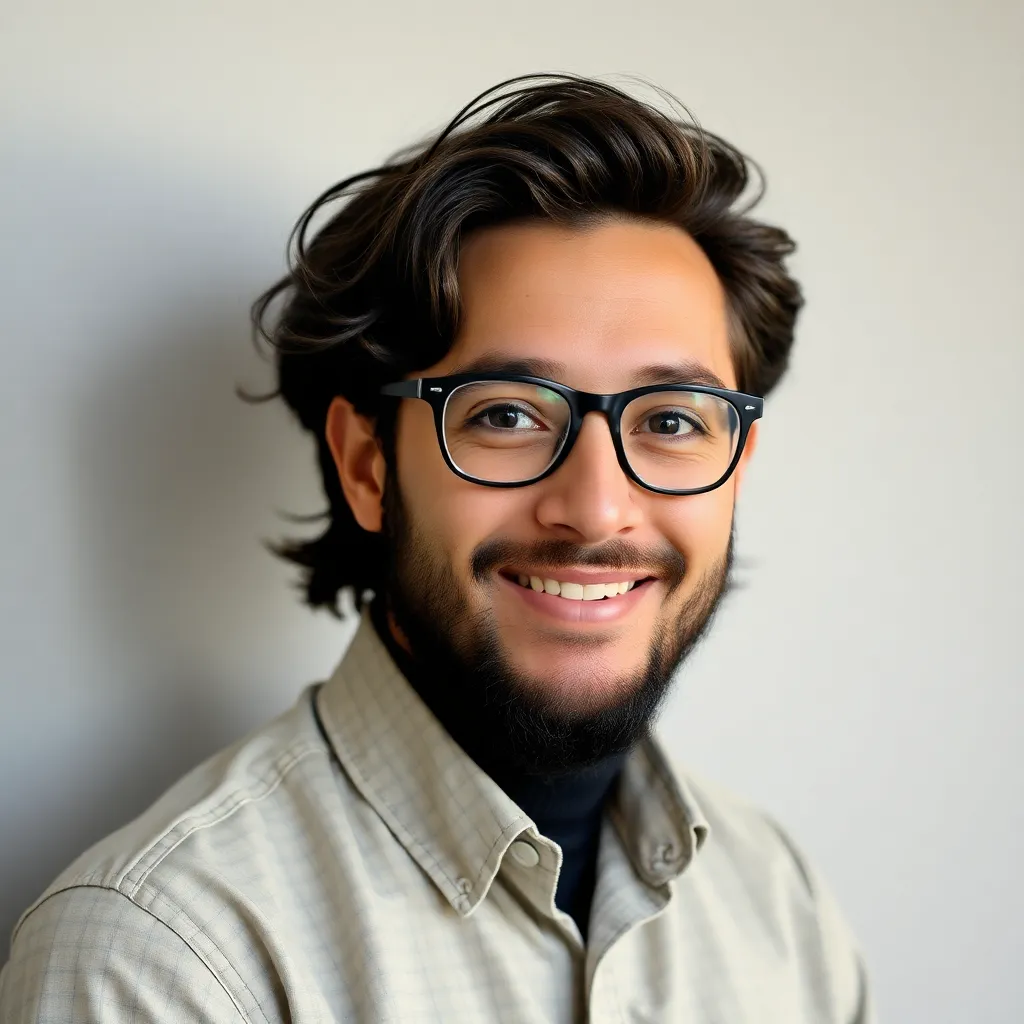
Breaking News Today
Apr 18, 2025 · 6 min read

Table of Contents
Creating an Automatic Basic Report from the Classes Table: A Comprehensive Guide
Generating reports automatically is crucial for efficient data management. This guide provides a step-by-step approach to creating a basic, automated report from a "Classes" table, covering various programming languages and methodologies. We'll explore different techniques, addressing common challenges and best practices to ensure your report is accurate, efficient, and easily understandable.
Understanding the "Classes" Table Structure
Before diving into the reporting process, let's define a hypothetical "Classes" table structure. This table could represent classes offered by an educational institution or training center. The fields might include:
- classID (INT, Primary Key): Unique identifier for each class.
- className (VARCHAR): Name of the class (e.g., "Introduction to Python," "Advanced SQL").
- instructorID (INT, Foreign Key): Links to an "Instructors" table (not covered in this example).
- startDate (DATE): Start date of the class.
- endDate (DATE): End date of the class.
- capacity (INT): Maximum number of students allowed.
- location (VARCHAR): Classroom or online platform where the class is held.
This structure will serve as our example, but the principles apply to other table designs.
Method 1: Using SQL for Direct Reporting
SQL (Structured Query Language) offers the most direct way to generate reports from databases. We can create a query that selects the necessary data and formats it appropriately.
Basic SQL Query
The simplest report might just list all classes:
SELECT classID, className, startDate, endDate, location
FROM Classes;
This query retrieves the class ID, name, start and end dates, and location. You can execute this query using any SQL client (like MySQL Workbench, pgAdmin, or SQL Developer) connected to your database. The results will be displayed in a tabular format.
Enhanced SQL Query with Calculations
We can enhance the query to include calculated fields, like the class duration:
SELECT classID, className, startDate, endDate, location,
JULIANDAY(endDate) - JULIANDAY(startDate) AS classDuration
FROM Classes;
This adds classDuration
, calculated as the difference between the Julian days of the start and end dates. Note that the specific function for calculating the difference might vary depending on your database system (e.g., DATEDIFF
in some systems).
SQL for Filtering and Sorting
To create more specific reports, you can add WHERE
clauses for filtering and ORDER BY
clauses for sorting. For example, to list only classes starting in 2024, ordered by start date:
SELECT classID, className, startDate, endDate, location
FROM Classes
WHERE strftime('%Y', startDate) = '2024'
ORDER BY startDate;
Method 2: Programmatic Reporting using Python
Python, with libraries like sqlite3
(for SQLite databases) or psycopg2
(for PostgreSQL), allows for more flexible report generation. You can fetch data from the database, manipulate it, and format it as needed.
Python Code Example (SQLite)
This example uses sqlite3
to connect to an SQLite database, fetch data, and print a formatted report:
import sqlite3
def generate_report(db_file):
conn = sqlite3.connect(db_file)
cursor = conn.cursor()
cursor.execute("SELECT classID, className, startDate, endDate, location FROM Classes")
rows = cursor.fetchall()
print("Classes Report:\n")
print("-------------------------------------------------------------------")
print("{:<10} {:<30} {:<15} {:<15} {:<20}".format("Class ID", "Class Name", "Start Date", "End Date", "Location"))
print("-------------------------------------------------------------------")
for row in rows:
print("{:<10} {:<30} {:<15} {:<15} {:<20}".format(row[0], row[1], row[2], row[3], row[4]))
conn.close()
generate_report('mydatabase.db') # Replace 'mydatabase.db' with your database file name
This code connects to the database, executes a query, fetches the results, and then prints them in a neatly formatted table using f-strings for formatting. Error handling and more sophisticated formatting could be added for robustness.
Python with Pandas for Data Manipulation
The pandas
library provides powerful data manipulation capabilities. It can read data from various sources, including databases, and allows for easy data cleaning, transformation, and report generation.
import sqlite3
import pandas as pd
def generate_pandas_report(db_file):
conn = sqlite3.connect(db_file)
df = pd.read_sql_query("SELECT * FROM Classes", conn)
conn.close()
#Data Manipulation Example: Calculate duration
df['duration'] = (pd.to_datetime(df['endDate']) - pd.to_datetime(df['startDate'])).dt.days
print(df.to_string(index=False)) #Prints the DataFrame as a formatted string.
generate_pandas_report('mydatabase.db')
This utilizes pandas.read_sql_query
for efficient data retrieval and leverages pandas' built-in capabilities for data manipulation and presentation.
Method 3: Using Reporting Tools
Specialized reporting tools offer advanced features for creating visually appealing and interactive reports. These tools often provide graphical interfaces, allowing for easy report design without extensive coding. Examples include:
- Tableau: A powerful business intelligence tool for data visualization and reporting.
- Power BI: Microsoft's business analytics service, offering similar capabilities to Tableau.
- JasperReports: An open-source reporting tool that can integrate with various databases and programming languages.
These tools typically involve connecting to your database, designing the report layout (choosing charts, tables, and other visualizations), and scheduling automated report generation.
Automating the Report Generation
To automate the report creation, you'll need to integrate the chosen method (SQL, Python, or reporting tool) into a scheduled task or script. The specifics depend on your operating system and environment:
- Scheduled Tasks (Windows): You can create a scheduled task that runs a batch script or Python script at specified intervals (daily, weekly, etc.).
- cron jobs (Linux/macOS):
cron
allows scheduling commands or scripts to run periodically. - Task Schedulers (Database Systems): Some database systems offer built-in task schedulers that can execute SQL queries or scripts automatically.
- Cloud Functions (AWS Lambda, Google Cloud Functions, Azure Functions): Cloud functions allow you to run code in response to events or on a schedule without managing servers.
The automation process generally involves:
- Connecting to the database: Establishing a connection to your database using appropriate credentials.
- Executing the report query or script: Running the SQL query or Python script to generate the report data.
- Formatting and saving the report: Formatting the output (e.g., into a CSV file, PDF, or HTML) and saving it to a designated location.
- Emailing the report (optional): Sending the generated report as an email attachment to relevant recipients.
Best Practices for Automated Reporting
- Error Handling: Implement robust error handling to catch and handle potential issues (e.g., database connection failures, query errors).
- Logging: Log important events (successful report generation, errors, warnings) for monitoring and troubleshooting.
- Security: Secure database credentials and protect the report generation process from unauthorized access.
- Maintainability: Write clean, well-documented code to ensure the report generation process is easy to maintain and update.
- Testing: Thoroughly test the automated report generation process to ensure accuracy and reliability.
- Data Validation: Implement data validation to ensure the data in your "Classes" table is accurate and consistent before generating the report.
Conclusion
Creating an automatic basic report from the "Classes" table involves choosing the appropriate method (SQL, Python, or reporting tools), implementing the necessary code or configuration, and automating the process using scheduled tasks or cloud functions. By following best practices and implementing robust error handling, you can create a reliable and maintainable system for generating accurate and timely reports. Remember to tailor the report's content and format to meet your specific needs and audience. This comprehensive guide provides a foundation for building more advanced and sophisticated automated reporting solutions as your requirements evolve.
Latest Posts
Latest Posts
-
When Engaging Stakeholders A Project Manager Should
Apr 19, 2025
-
Nj Real Estate Exam Questions Pdf Free
Apr 19, 2025
-
Operating Systems Like Windows And Macos Are Single User Single Task Oss
Apr 19, 2025
-
What Condition Is Most Necessary To Build A Glacier
Apr 19, 2025
-
Angle Measures And Segment Lengths Quick Check
Apr 19, 2025
Related Post
Thank you for visiting our website which covers about Create An Automatic Basic Report From The Classes Table . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.