Express The Group Number As An Integer
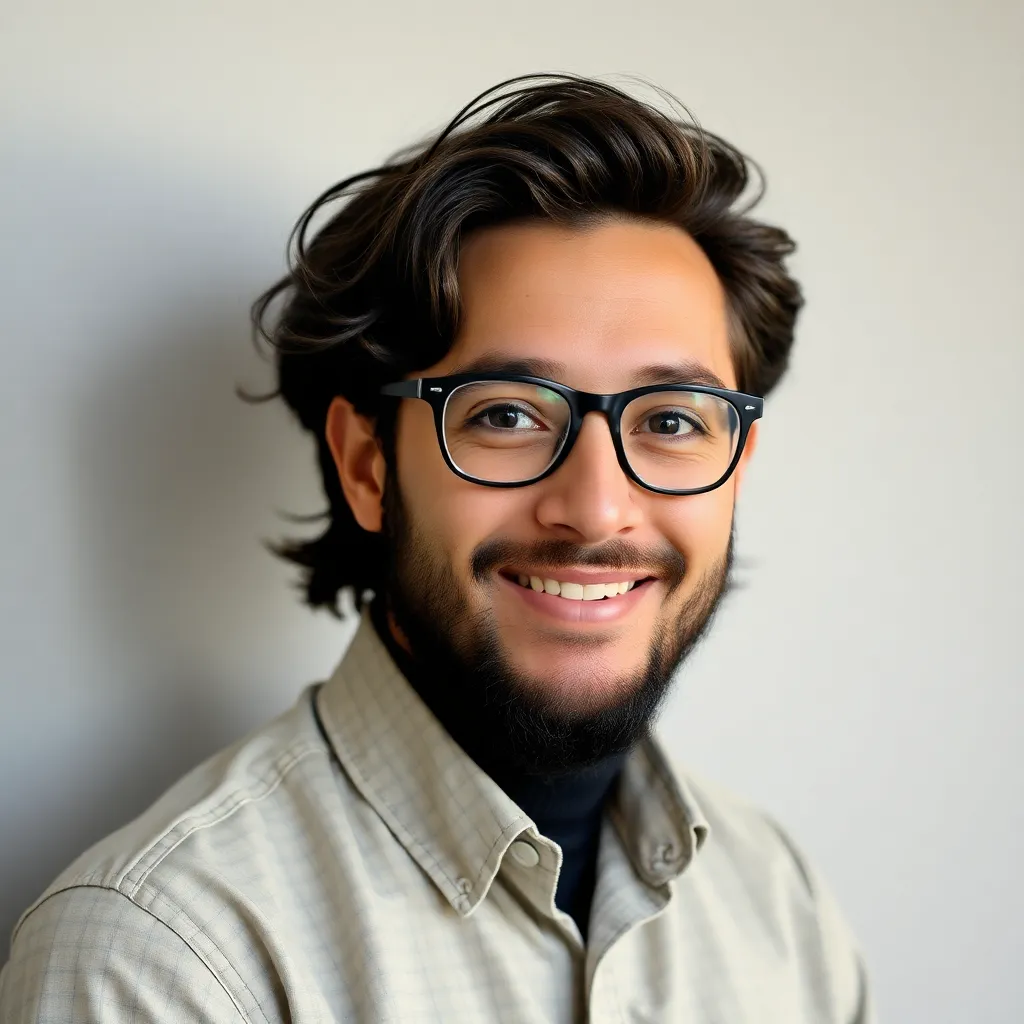
Breaking News Today
May 09, 2025 · 6 min read

Table of Contents
Expressing the Group Number as an Integer: A Comprehensive Guide
Representing group numbers as integers is a fundamental concept in programming and data management. This seemingly simple task can become surprisingly complex when dealing with various data structures, potential edge cases, and the need for efficient and robust solutions. This article delves deep into the intricacies of expressing group numbers as integers, exploring different approaches, addressing potential challenges, and providing practical examples to solidify your understanding.
Understanding the Problem: Why Integers?
Before diving into the solutions, let's establish the "why." Why do we need to express group numbers as integers? The primary reasons include:
-
Efficiency: Integers are the most efficient data type for representing numerical quantities. They require less memory compared to strings or other complex data structures. This is crucial when dealing with large datasets or resource-constrained environments.
-
Comparability: Integers allow for easy comparison and sorting. Determining the order of groups becomes trivial when represented numerically. This is essential for tasks such as ranking, filtering, and data analysis.
-
Mathematical Operations: Integers can be used directly in mathematical calculations. This enables sophisticated analyses and manipulations of group data, such as calculating averages, sums, or differences between groups.
-
Database Integration: Many database systems optimize the storage and retrieval of integer data. Representing group numbers as integers improves database performance and simplifies data management.
-
Algorithm Compatibility: Many algorithms and data structures rely on integer indexing or ordering. Using integer group numbers ensures seamless integration with existing tools and techniques.
Methods for Expressing Group Numbers as Integers
The most appropriate method for converting group numbers to integers depends heavily on the nature of the group numbers themselves. Let's explore some common scenarios and their corresponding solutions:
1. Sequential Numbering: The Simplest Approach
This is the most straightforward method when group numbers are already inherently sequential or can be easily made so. For instance, if your groups are labeled as "Group A," "Group B," "Group C," etc., you can simply assign them integer values:
- Group A: 1
- Group B: 2
- Group C: 3
- ...and so on.
This approach is ideal for situations where the order of groups is significant and the number of groups is relatively small. The implementation is trivial:
group_names = ["Group A", "Group B", "Group C", "Group D"]
group_numbers = list(range(1, len(group_names) + 1))
group_mapping = dict(zip(group_names, group_numbers))
print(group_mapping) # Output: {'Group A': 1, 'Group B': 2, 'Group C': 3, 'Group D': 4}
2. Handling Non-Sequential Group Numbers: Enumeration or Mapping
If your group numbers are not sequentially labeled (e.g., "Group X1," "Group Y3," "Group Z2"), you need a more sophisticated approach. This typically involves creating a mapping between the original group names and their corresponding integer representations.
This can be done using dictionaries (Python) or hash maps (other languages):
group_names = ["Group X1", "Group Y3", "Group Z2"]
group_numbers = [1, 2, 3] # Assign arbitrary integer values
group_mapping = dict(zip(group_names, group_numbers))
print(group_mapping) # Output: {'Group X1': 1, 'Group Y3': 2, 'Group Z2': 3}
# Accessing integer representation:
print(group_mapping["Group Y3"]) # Output: 2
Important Note: When using arbitrary integer assignments, it’s crucial to maintain consistency throughout your data processing pipeline.
3. Utilizing Ordinal Encoding (for categorical data):
If your groups are categorical variables, ordinal encoding is a suitable technique. This involves assigning integers to each unique group based on their order of appearance in the data. Libraries like scikit-learn in Python provide efficient functions for this purpose.
However, be cautious: this method implicitly assumes an ordinal relationship between groups. If no such relationship exists, ordinal encoding might introduce unintended bias into your analysis.
4. Advanced Techniques for Large Datasets: Hashing
For very large datasets with a vast number of unique group names, direct mapping might become inefficient in terms of memory usage. In such cases, hashing functions can provide a more scalable solution. Hash functions map arbitrary-length input (group names) to fixed-size output (integer representations). While collisions (multiple group names mapping to the same integer) are possible, efficient hash functions minimize this risk.
Addressing Challenges and Potential Pitfalls
While expressing group numbers as integers is generally straightforward, several potential challenges need careful consideration:
-
Data Integrity: Ensure your mapping is consistent and accurate. Errors in the mapping can lead to incorrect analyses and flawed conclusions.
-
Collision Handling: When using hashing, carefully address the possibility of collisions. Employ techniques such as chaining or open addressing to manage collisions effectively.
-
Scalability: For large datasets, choose methods that scale efficiently. Avoid methods that require excessive memory or processing time.
-
Data Type Considerations: Ensure the integer data type used is sufficient to represent all group numbers. For example, if you have more than 2,147,483,647 groups, you'll need a 64-bit integer data type (long long in C++ or long in Java).
Practical Examples and Code Snippets
Let's illustrate the concepts with more elaborate examples:
Example 1: Sequential Numbering with Error Handling:
def assign_sequential_numbers(group_names):
"""Assigns sequential integer numbers to group names. Handles duplicates."""
group_mapping = {}
next_number = 1
for name in group_names:
if name not in group_mapping:
group_mapping[name] = next_number
next_number += 1
return group_mapping
group_names = ["Group A", "Group B", "Group C", "Group A", "Group D"]
mapping = assign_sequential_numbers(group_names)
print(mapping) #Output: {'Group A': 1, 'Group B': 2, 'Group C': 3, 'Group D': 4}
Example 2: Mapping using a CSV file:
Imagine you have a CSV file containing group names and their corresponding descriptions. You could use Python's csv
module to read the file and create a mapping:
import csv
def create_mapping_from_csv(filepath):
mapping = {}
with open(filepath, 'r', newline='') as csvfile:
reader = csv.reader(csvfile)
next(reader) # Skip header row (if any)
for row in reader:
group_name = row[0] #Assuming group name is in the first column. Adjust as needed.
try:
group_number = int(row[1]) #Assuming group number is in the second column
mapping[group_name] = group_number
except (ValueError, IndexError):
print(f"Error processing row: {row}. Skipping.")
return mapping
This example highlights the importance of robust error handling. The try-except
block prevents crashes if the CSV file is malformed.
Conclusion: Choosing the Right Approach
Selecting the best method for expressing group numbers as integers depends on factors such as the nature of your data, the size of your dataset, and the complexity of your analysis. Carefully consider the potential challenges and implement robust error handling to ensure the accuracy and efficiency of your solution. This comprehensive guide provides a strong foundation for tackling this fundamental data processing task effectively. Remember to always prioritize data integrity and scalability when dealing with large-scale data processing. By understanding the various techniques and their limitations, you can choose the optimal approach for your specific needs.
Latest Posts
Latest Posts
-
How Do Plot Events Affect Ophelias Character Development
May 11, 2025
-
America The Story Of Us Bust Episode 9
May 11, 2025
-
When Transporting A Stable Stroke Patient With A Paralyzed Extremity
May 11, 2025
-
Healthcare Quality In The United States Is Characterized By Quizlet
May 11, 2025
-
The Results Of A Scientific Experiment Are Called Blank
May 11, 2025
Related Post
Thank you for visiting our website which covers about Express The Group Number As An Integer . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.