Indexing Works With Both Strings And Lists
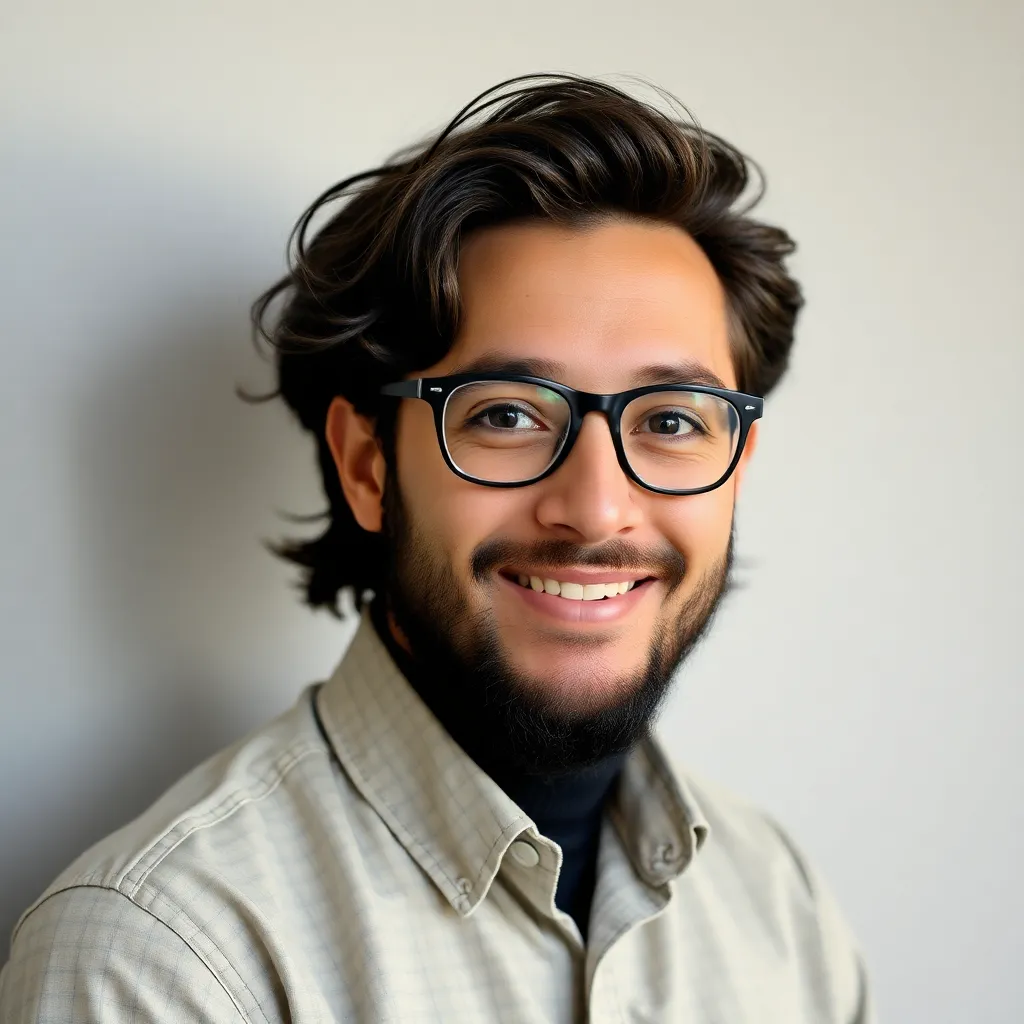
Breaking News Today
May 09, 2025 · 7 min read
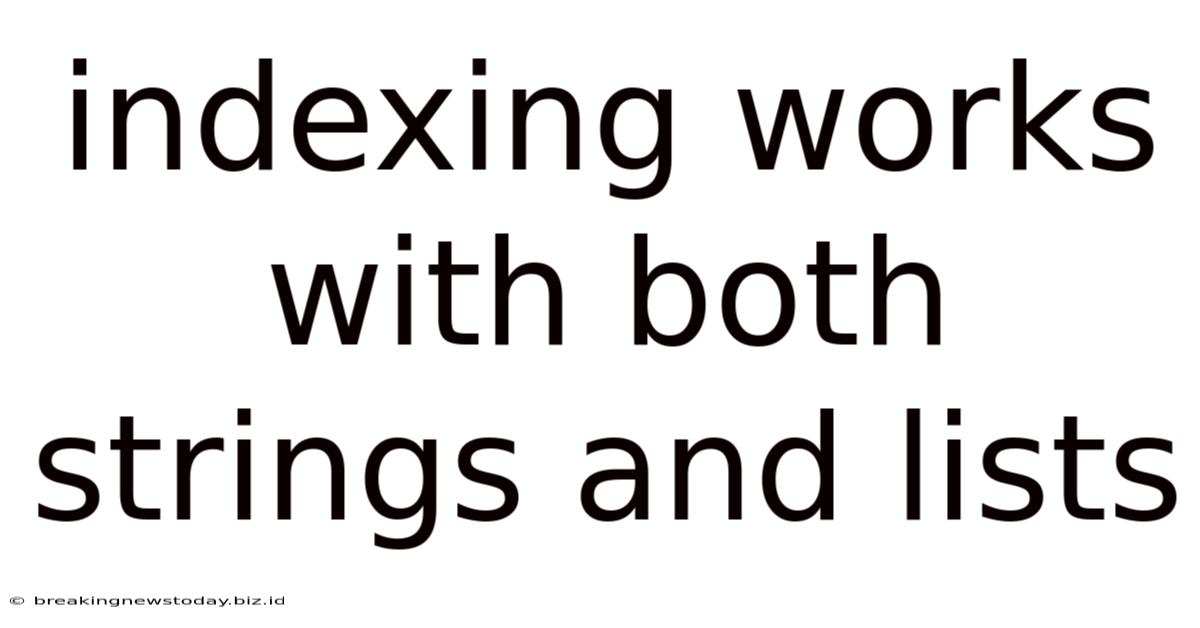
Table of Contents
Indexing Works with Both Strings and Lists: A Deep Dive
Indexing is a fundamental concept in computer science, crucial for accessing and manipulating data within various data structures. While often introduced with arrays or lists, its application extends seamlessly to strings, which are essentially ordered sequences of characters. Understanding how indexing functions with both strings and lists is paramount for effective programming in any language. This comprehensive guide will delve into the intricacies of indexing, comparing and contrasting its use with strings and lists, and exploring its practical applications.
Understanding Indexing: The Foundation
Indexing provides a mechanism to access individual elements within an ordered collection of data. This "ordered collection" can manifest as a list, an array, a string, or even more complex data structures. Each element within the collection is assigned a numerical index, typically starting from 0 (zero-based indexing) – a convention adopted by many popular programming languages like Python, Java, and JavaScript. This means the first element has an index of 0, the second element has an index of 1, and so on.
Key Differences between Strings and Lists:
While both strings and lists employ indexing, their fundamental nature differentiates how indexing operates:
-
Strings: Represent sequences of characters. They are immutable, meaning their contents cannot be changed after creation. Indexing a string retrieves the character at the specified index.
-
Lists: Are mutable ordered collections of items. The items can be of diverse data types (integers, strings, floats, other lists, etc.). Indexing a list retrieves the item at the specified index, and the value at that index can be modified.
Indexing Strings: Character Access
In many programming languages, strings behave like arrays of characters. Indexing a string allows you to extract individual characters, substrings, or perform character-by-character operations. Let's explore this with examples in Python:
my_string = "Hello, World!"
# Accessing individual characters
print(my_string[0]) # Output: H
print(my_string[7]) # Output: W
# Accessing a substring (slicing)
print(my_string[7:12]) # Output: World
# Iterating through the string using indices
for i in range(len(my_string)):
print(my_string[i])
This code demonstrates how to access individual characters and substrings using indexing and slicing. Slicing utilizes two indices: a start index (inclusive) and an end index (exclusive). The len()
function returns the length of the string, crucial for iterating through all characters. Remember, attempting to access an index beyond the string's length will result in an IndexError
.
String Manipulation through Indexing:
Indexing is fundamental to numerous string manipulation tasks:
- Reversing a string: Iterating through the string from the last index to the first.
- Finding character occurrences: Checking characters at each index for a match.
- Replacing characters: Modifying the string (in languages that allow mutable strings, though Python strings are immutable, requiring creation of a new string).
- Palindrome checks: Comparing characters at symmetrical indices.
Indexing Lists: Item Retrieval and Modification
Lists provide more flexibility than strings due to their mutable nature and ability to store heterogeneous data types. Indexing a list allows you to retrieve, modify, or delete individual elements.
my_list = [10, "apple", 3.14, True, [1, 2, 3]]
# Accessing elements
print(my_list[0]) # Output: 10
print(my_list[1]) # Output: apple
# Modifying elements
my_list[0] = 20
print(my_list) # Output: [20, "apple", 3.14, True, [1, 2, 3]]
# Deleting elements
del my_list[3]
print(my_list) # Output: [20, "apple", 3.14, [1, 2, 3]]
This illustrates how to access, modify, and delete elements using their indices. The mutability of lists is a key distinction from strings. Similar to strings, exceeding the list's index bounds leads to an IndexError
.
List Manipulation Using Indexing:
Indexing empowers various list operations:
- Sorting lists: Algorithms like bubble sort or merge sort heavily rely on index-based comparisons and swaps.
- Searching lists: Linear search uses indexing to traverse the list and find a specific element.
- Inserting and deleting elements: Efficiently adding or removing elements at specific positions.
- Nested list manipulation: Accessing elements within lists contained within other lists.
Negative Indexing: A Powerful Tool
Both strings and lists support negative indexing. Negative indices count backward from the end of the sequence. The last element has an index of -1, the second-to-last element has an index of -2, and so on.
my_string = "Python"
print(my_string[-1]) # Output: n
print(my_string[-3]) # Output: h
my_list = [1, 2, 3, 4, 5]
print(my_list[-1]) # Output: 5
print(my_list[-2]) # Output: 4
Negative indexing simplifies accessing the last few elements of a sequence without needing to calculate the exact positive index. It's particularly useful when dealing with sequences of unknown length.
Slicing: Extracting Subsequences
Slicing allows extracting a portion (subsequence) of a string or list. It uses a colon (:
) to specify a range of indices. The syntax is generally [start:end:step]
, where:
start
: The starting index (inclusive). Defaults to 0 if omitted.end
: The ending index (exclusive). Defaults to the end of the sequence if omitted.step
: The increment between indices. Defaults to 1 if omitted.
my_string = "abcdefg"
print(my_string[2:5]) # Output: cde
print(my_string[::2]) # Output: aceg (every other character)
print(my_string[::-1]) # Output: gfedcba (reversed string)
my_list = [10, 20, 30, 40, 50]
print(my_list[1:4]) # Output: [20, 30, 40]
print(my_list[::-1]) # Output: [50, 40, 30, 20, 10] (reversed list)
Slicing provides concise ways to extract subsequences, reverse sequences, or extract elements with specific intervals.
Multidimensional Indexing: Nested Lists and Matrices
Indexing extends to multidimensional data structures like nested lists (which can represent matrices or arrays). Accessing elements requires multiple indices, one for each dimension.
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Accessing element at row 1, column 2
print(matrix[1][2]) # Output: 6
# Iterating through the matrix
for i in range(len(matrix)):
for j in range(len(matrix[i])):
print(matrix[i][j])
This demonstrates how to access individual elements in a nested list using multiple indices. The first index refers to the row, and the second index refers to the column. Iteration through multidimensional structures often uses nested loops.
Error Handling: Preventing IndexErrors
Attempting to access an index outside the bounds of a string or list raises an IndexError
. Robust code includes error handling to gracefully manage these situations.
my_list = [1, 2, 3]
try:
print(my_list[3])
except IndexError:
print("Index out of bounds!")
This try-except
block prevents the program from crashing due to an IndexError
. Instead, it prints an informative message.
Performance Considerations: Optimization Strategies
For large strings or lists, indexing operations are generally efficient with a time complexity of O(1) – constant time. However, certain operations, like slicing large portions of a list, can have performance implications. Optimized approaches include:
- Using generators: For large datasets, generating items on demand rather than creating a complete copy in memory can improve efficiency.
- Vectorized operations: Libraries like NumPy (for numerical data) offer optimized functions that perform operations on entire arrays without explicit indexing loops, significantly speeding up computations.
Real-world Applications of Indexing
Indexing finds extensive application across numerous areas:
- Data analysis: Accessing specific data points in datasets, performing calculations on subsets of data, and manipulating data structures.
- Text processing: Extracting keywords, manipulating substrings, searching for patterns in text documents.
- Image processing: Accessing pixel values within an image represented as a multi-dimensional array.
- Database management: Retrieving data from databases efficiently using indexed fields.
- Game development: Managing game state, accessing game objects, and performing collision detection.
- Machine learning: Accessing and manipulating features in datasets for model training and prediction.
- Web development: Processing user input, extracting data from forms, and manipulating strings.
Conclusion: Mastery of Indexing for Efficient Programming
Indexing is a foundational concept essential for manipulating strings and lists efficiently. Understanding how indexing works, including negative indexing, slicing, and multidimensional indexing, is vital for writing effective and efficient code. By incorporating appropriate error handling and considering performance optimizations, you can leverage indexing to build robust and scalable applications across various domains. Mastering indexing empowers you to work with data structures effectively, leading to cleaner, more maintainable, and more performant code.
Latest Posts
Latest Posts
-
Which Of The Following Does Not Influence Perception
May 09, 2025
-
Select The Account Classification That Matches With The Description
May 09, 2025
-
Unit 8 Test Study Guide Quadratic Equations
May 09, 2025
-
After 4 Minutes Of Rescue Breathing No Pulse
May 09, 2025
-
The Most Abused Psychotropic Drug In The United States Is
May 09, 2025
Related Post
Thank you for visiting our website which covers about Indexing Works With Both Strings And Lists . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.