Introduction To Algorithms Fourth Edition Exercise Solutions
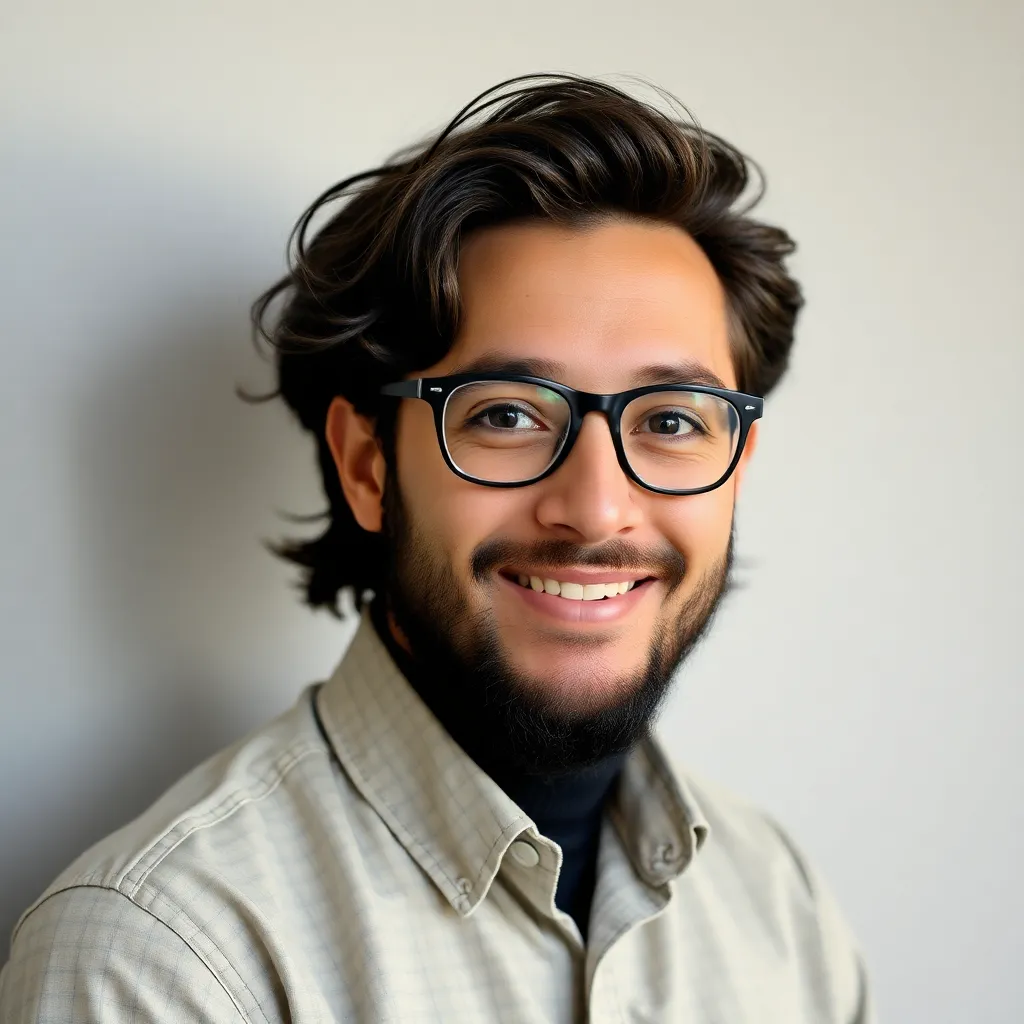
Breaking News Today
Mar 30, 2025 · 7 min read
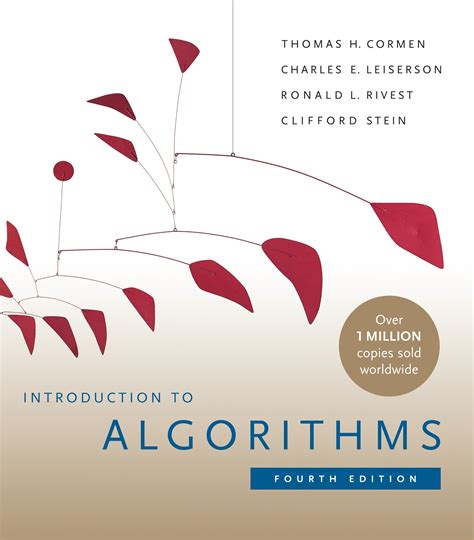
Table of Contents
Introduction to Algorithms, Fourth Edition: Exercise Solutions – A Comprehensive Guide
This comprehensive guide delves into the exercise solutions for Introduction to Algorithms, Fourth Edition, by Cormen, Leiserson, Rivest, and Stein (CLRS). We'll explore a selection of problems, focusing on the core concepts and providing detailed explanations to enhance your understanding. This isn't a simple answer sheet; it's a learning experience designed to solidify your grasp of algorithmic design and analysis. Remember, the true value lies not just in the solutions themselves, but in the process of arriving at them.
Chapter 2: Getting Started
This chapter lays the groundwork for the rest of the book. While many exercises are relatively straightforward, they serve as crucial warm-ups and reinforce fundamental concepts.
Exercise 2.1-1: Analyzing Insertion Sort
Problem: Illustrate the operation of INSERTION-SORT on the array A = [31, 41, 59, 26, 41, 58].
Solution: Insertion sort iteratively builds a sorted subarray. Let's trace the steps:
- A = [31, 41, 59, 26, 41, 58] (Initially unsorted)
- A = [31, 41, 59, 26, 41, 58] (31 is already sorted)
- A = [31, 41, 59, 26, 41, 58] (41 is compared to 31 and placed correctly)
- A = [31, 41, 59, 26, 41, 58] (59 is compared to 41 and 31, and placed correctly)
- A = [26, 31, 41, 59, 41, 58] (26 is compared to 59, 41, and 31, then inserted at the beginning)
- A = [26, 31, 41, 41, 59, 58] (The second 41 is inserted into its correct position)
- A = [26, 31, 41, 41, 58, 59] (58 is compared and inserted)
The final sorted array is [26, 31, 41, 41, 58, 59]. This exercise highlights the iterative nature of insertion sort and its simplicity.
Exercise 2.1-3: Loop Invariants
Problem: Give a careful argument that the correctness of the algorithm for insertion sort follows from the loop invariant.
Solution: This requires a formal proof using loop invariants. The loop invariant is usually structured as follows:
- Initialization: Show that the invariant holds before the first iteration of the loop.
- Maintenance: Show that if the invariant holds before an iteration, it holds after the iteration.
- Termination: Show that when the loop terminates, the invariant, along with the condition that caused the loop to terminate, gives a useful property that helps show that the algorithm is correct.
For insertion sort, a suitable loop invariant might be: "At the start of each iteration of the outer loop, the subarray A[1..j-1] consists of the elements originally in A[1..j-1], but in sorted order." Proving this invariant holds through the three stages demonstrates the correctness of the algorithm.
Exercise 2.2-2: Analyzing Merge Sort
Problem: Write pseudocode for MERGE-SORT that sorts a subarray A[p..r].
Solution: MERGE-SORT recursively divides the array until subarrays of size 1 (which are inherently sorted) are reached. Then, it merges the sorted subarrays.
MERGE-SORT(A, p, r)
if p < r
q = floor((p+r)/2)
MERGE-SORT(A, p, q)
MERGE-SORT(A, q+1, r)
MERGE(A, p, q, r)
The MERGE
procedure efficiently merges two sorted subarrays. This exercise emphasizes the divide-and-conquer strategy inherent in merge sort.
Chapter 3: Growth of Functions
This chapter introduces Big O notation and related concepts, crucial for analyzing algorithm efficiency.
Exercise 3.1-1: Big O Notation
Problem: Rank the following functions by order of growth: lg n, √n, n, n lg n, n², 2<sup>n</sup>, n<sup>n</sup>.
Solution: The correct ranking in increasing order of growth is: lg n, √n, n, n lg n, n², 2<sup>n</sup>, n<sup>n</sup>. This exercise reinforces the understanding of different growth rates and their relative dominance.
Exercise 3.2-4: Asymptotic Notation Properties
Problem: Prove that if f(n) = Θ(g(n)), then f(n) = O(g(n)).
Solution: This involves understanding the definitions of Θ and O notation. If f(n) = Θ(g(n)), it means there exist positive constants c<sub>1</sub>, c<sub>2</sub>, and n<sub>0</sub> such that 0 ≤ c<sub>1</sub>g(n) ≤ f(n) ≤ c<sub>2</sub>g(n) for all n ≥ n<sub>0</sub>. This directly implies that f(n) ≤ c<sub>2</sub>g(n) for all n ≥ n<sub>0</sub>, which is the definition of f(n) = O(g(n)). This proves the statement.
Chapter 4: Divide and Conquer
This chapter delves into the divide-and-conquer paradigm, illustrated prominently by merge sort and other algorithms.
Exercise 4.1-1: Recursive Algorithms
Problem: Describe a recursive algorithm to compute the factorial of a positive integer.
Solution:
FACTORIAL(n)
if n == 0
return 1
else
return n * FACTORIAL(n-1)
This simple recursive algorithm clearly demonstrates the divide-and-conquer approach, reducing the problem to smaller subproblems until the base case is reached.
Exercise 4.2-3: Strassen's Algorithm
Problem: Give an example of two 2 x 2 matrices whose product cannot be computed using fewer than 7 multiplications.
Solution: This requires careful consideration of Strassen's algorithm and its optimization of matrix multiplication. Constructing two matrices that defy any further optimization requires exploring the limitations of the algorithm's underlying mathematical properties. Finding such an example involves a deeper understanding of linear algebra and its connections to algorithmic complexity. This isn't a trivial task and often requires exploring different matrix combinations.
Chapter 5: Probabilistic Analysis and Randomized Algorithms
This chapter introduces probabilistic analysis and randomized algorithms, adding a new dimension to algorithm design.
Exercise 5.1-1: Expected Value
Problem: What is the expected value of a random variable X that is 1 with probability p and 0 with probability 1 - p?
Solution: E[X] = (1)(p) + (0)(1-p) = p. This straightforward exercise establishes the fundamental concept of expected value in a simple context.
Exercise 5.2-1: Randomized Algorithms
Problem: Describe an algorithm that, given n integers in an array, finds the kth smallest integer in expected O(n) time.
Solution: This problem points towards the use of randomized selection, often employing a randomized partition similar to quicksort. The algorithm involves randomly selecting a pivot element, partitioning the array based on the pivot, and recursively processing either the lower or upper partition depending on the position of the pivot relative to k. The expected O(n) time complexity stems from the probabilistic properties of the randomized pivot selection.
Chapter 6: Heapsort
This chapter introduces heapsort, an efficient in-place sorting algorithm.
Exercise 6.1-1: Max-Heaps
Problem: Draw the binary tree corresponding to the following max-heap: [15, 13, 9, 5, 12, 8, 7, 4, 0, 6, 11, 3, 2, 1]
Solution: This exercise involves visually representing the heap as a binary tree, ensuring that the parent nodes always have values greater than their children (the max-heap property). Drawing this tree illustrates the heap's structure and its relationship to the array representation.
Exercise 6.2-6: Heap Operations
Problem: Show how to implement HEAP-EXTRACT-MAX in O(lg n) time.
Solution: HEAP-EXTRACT-MAX involves removing the root (maximum) element. Replacing it with the last element and then calling MAX-HEAPIFY to restore the heap property guarantees the O(lg n) time complexity due to the logarithmic nature of MAX-HEAPIFY's traversal down the heap.
Conclusion
This exploration of select exercises from Introduction to Algorithms, Fourth Edition, provides a foundation for understanding fundamental algorithmic concepts. Remember, the journey through these problems is as important as the solutions themselves. Grappling with these challenges strengthens your problem-solving skills and reinforces your understanding of algorithmic design, analysis, and efficiency. The true mastery of algorithms comes through practice and persistent engagement. Continue to explore the remaining exercises in the book to deepen your expertise in this vital field of computer science.
Latest Posts
Latest Posts
-
All Of The Following People Should Receive W 2 Forms Except
Apr 01, 2025
-
Nurse Logic Knowledge And Clinical Judgment Beginner
Apr 01, 2025
-
First 36 Elements On The Periodic Table
Apr 01, 2025
-
A Computer Training Business Needs To Hire A Consultant
Apr 01, 2025
-
All Results Have An Obvious Link To A Landing Page
Apr 01, 2025
Related Post
Thank you for visiting our website which covers about Introduction To Algorithms Fourth Edition Exercise Solutions . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.