Manipulators Without Parameters Are Part Of The ____ Header File.
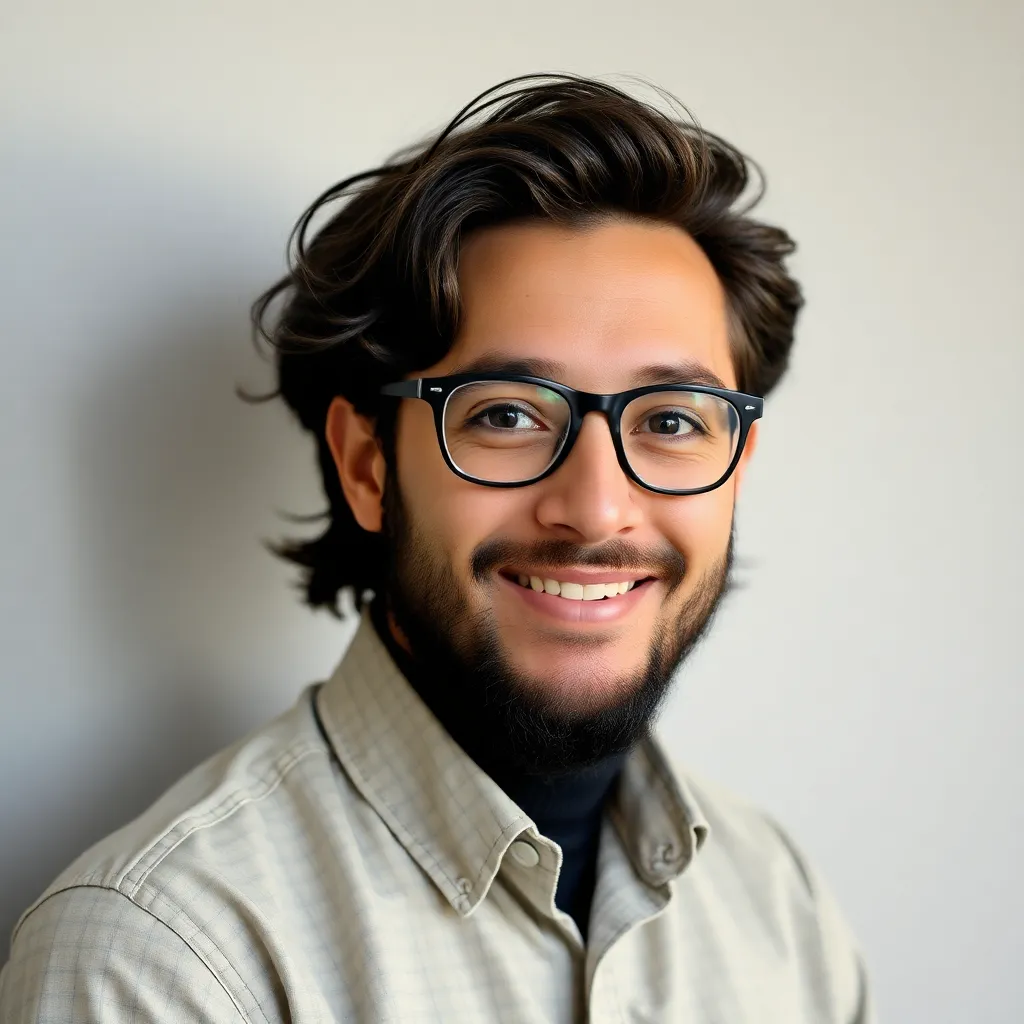
Breaking News Today
Mar 13, 2025 · 5 min read

Table of Contents
Manipulators Without Parameters are Part of the <iostream>
Header File
Manipulators are powerful tools in C++ that allow you to modify the behavior of input and output streams. They offer a flexible and elegant way to control formatting, ensuring your data is presented clearly and consistently. Understanding manipulators is crucial for writing clean, efficient, and readable C++ code, especially when working with standard input/output streams. This comprehensive guide delves into the world of manipulators, focusing specifically on those without parameters and their association with the <iostream>
header file.
What are Manipulators?
Manipulators are essentially functions that operate on input/output streams (like cout
and cin
). They alter the stream's state, affecting how subsequent data is read or written. This includes changing formatting aspects like the number of decimal places displayed, aligning text, or setting the output base (decimal, hexadecimal, octal). They are invoked using the insertion (<<
) and extraction (>>
) operators, seamlessly integrating into your stream operations.
The <iostream>
Header File: The Heart of I/O
The <iostream>
header file is fundamental to C++'s input/output capabilities. It defines essential classes like istream
(input stream) and ostream
(output stream), along with the standard streams cin
(standard input), cout
(standard output), and cerr
(standard error). Crucially, it also declares many built-in manipulators, including those that don't require any parameters. These parameterless manipulators offer quick and efficient ways to adjust stream behavior.
Parameterless Manipulators: A Closer Look
Parameterless manipulators simplify common formatting tasks. They don't require you to pass any arguments; their function is predefined. This makes them particularly convenient for frequently used formatting adjustments. Let's explore some of the key parameterless manipulators declared within the <iostream>
header:
1. endl
(End Line):
This is arguably the most commonly used manipulator. endl
inserts a newline character into the output stream and then flushes the buffer. Flushing ensures that all data written to the stream is immediately sent to the output device (e.g., the console or a file). This is important for situations where you need immediate output, such as displaying progress updates in a long-running program.
#include
int main() {
std::cout << "Hello" << std::endl;
std::cout << "World!"; // Newline from endl ensures these are on separate lines.
return 0;
}
2. flush
:
The flush
manipulator forces the output buffer to be flushed. Unlike endl
, it doesn't insert a newline character. Use flush
when you need to guarantee that the buffered output is sent without waiting for the buffer to fill or a newline. This is particularly relevant in situations requiring real-time updates or when dealing with potentially unstable output streams.
#include
int main() {
std::cout << "Progress: " << 50 << "%" << std::flush; // Immediate output update
// ...some time-consuming operation...
std::cout << " Complete!" << std::endl;
return 0;
}
3. ws
(Whitespace):
The ws
manipulator is used with input streams (cin
). It extracts and discards leading whitespace characters (spaces, tabs, newlines) from the input stream. This is incredibly useful for cleaning up user input before parsing or processing numerical data.
#include
int main() {
int age;
std::cout << "Enter your age: ";
std::cin >> std::ws >> age; // discards leading whitespace
std::cout << "You are " << age << " years old." << std::endl;
return 0;
}
4. dec
, hex
, and oct
(Base Conversion):
These manipulators control the base (radix) used for outputting integers. dec
sets the base to decimal (base 10), hex
sets it to hexadecimal (base 16), and oct
sets it to octal (base 8). This allows you to easily display integers in different representations.
#include
#include // needed for setw
int main() {
int num = 255;
std::cout << "Decimal: " << std::dec << num << std::endl;
std::cout << "Hexadecimal: " << std::hex << num << std::endl;
std::cout << "Octal: " << std::oct << num << std::endl;
return 0;
}
Note that iomanip
is necessary for manipulators that require extra functionality like setting the width.
Why Use Parameterless Manipulators?
The elegance of parameterless manipulators lies in their simplicity. They are easy to use, improving code readability. Their inherent conciseness also makes them ideal for enhancing the flow of your code. Furthermore, their inclusion within the <iostream>
header ensures they are readily available and universally recognized within the C++ standard library.
Advanced Usage and Considerations
While parameterless manipulators are efficient for basic formatting, many situations demand more fine-grained control. For such cases, parameterized manipulators from <iomanip>
(like setw
, setprecision
, setfill
) are essential. These manipulators take arguments to specify the desired formatting details. Using a combination of parameterless and parameterized manipulators allows for precise and adaptable output formatting.
Example combining parameterless and parameterized manipulators:
#include
#include
int main() {
double pi = 3.14159265359;
std::cout << "Pi (to 3 decimal places): " << std::setprecision(3) << pi << std::endl;
std::cout << std::setw(10) << std::setfill('*') << "Formatted" << std::endl;
return 0;
}
Custom Manipulators: Extending Functionality
For truly specialized formatting needs, you can define your own manipulators. This involves creating functions that take an ostream
or istream
as an argument and modify its state accordingly. This level of customization grants ultimate control over how your input/output streams behave.
Best Practices for Using Manipulators
-
Choose the Right Manipulator: Select the manipulator that best suits the specific formatting requirement. Parameterless manipulators offer a quick solution for common tasks, while parameterized ones provide fine-grained control.
-
Consistency is Key: Maintain consistent formatting throughout your code for better readability and maintainability.
-
Avoid Overuse: While manipulators are powerful, don't overuse them unnecessarily. Excessive manipulation can complicate the code and make it harder to understand.
-
Testing: Thoroughly test your code with various inputs and outputs to ensure manipulators behave as intended.
Conclusion: Mastering Manipulators for Elegant I/O
Manipulators are an integral part of effective C++ input/output operations. Understanding parameterless manipulators, readily available in the <iostream>
header, empowers you to streamline your code and produce clean, well-formatted output. By mastering both parameterless and parameterized manipulators, along with potentially creating custom manipulators, you can achieve precise control over your input/output operations, ultimately improving your C++ programming proficiency. The key is to choose the right tool for the job and strive for consistent, clear, and readable code. This comprehensive understanding of manipulators contributes significantly to writing high-quality, efficient, and maintainable C++ applications. Remember that effective use of manipulators enhances not only the appearance of your output but also the overall clarity and elegance of your code.
Latest Posts
Latest Posts
-
The Core Element Of Every Play Is
May 09, 2025
-
During The Meuse Argonne Offensive Of 1918 The Americans Helped
May 09, 2025
-
What Can Cause Secondary Brain Injury Pals
May 09, 2025
-
Why Does Proctor Refuse To Sign The Confession
May 09, 2025
-
People Benefit From Talking About Their Problems
May 09, 2025
Related Post
Thank you for visiting our website which covers about Manipulators Without Parameters Are Part Of The ____ Header File. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.