Match Each Language Concept With The Appropriate Example.
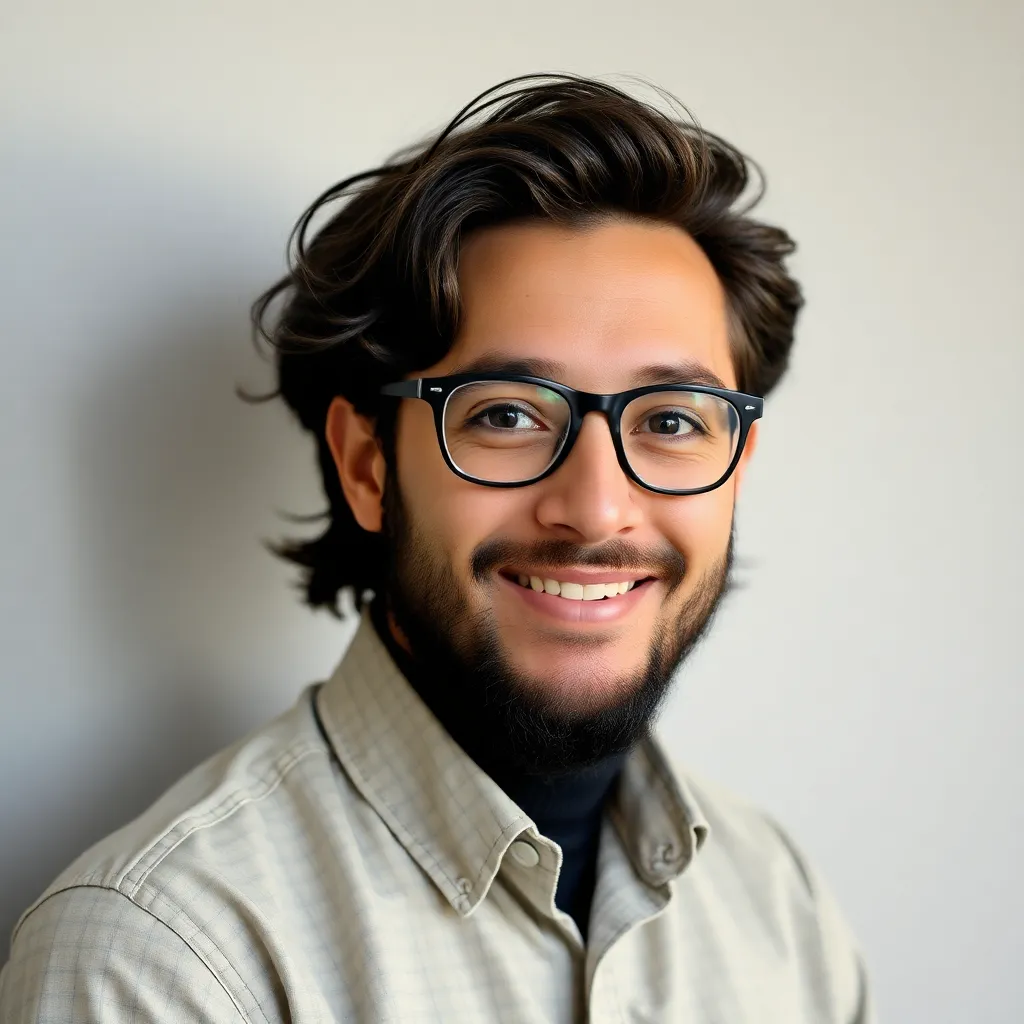
Breaking News Today
May 12, 2025 · 6 min read
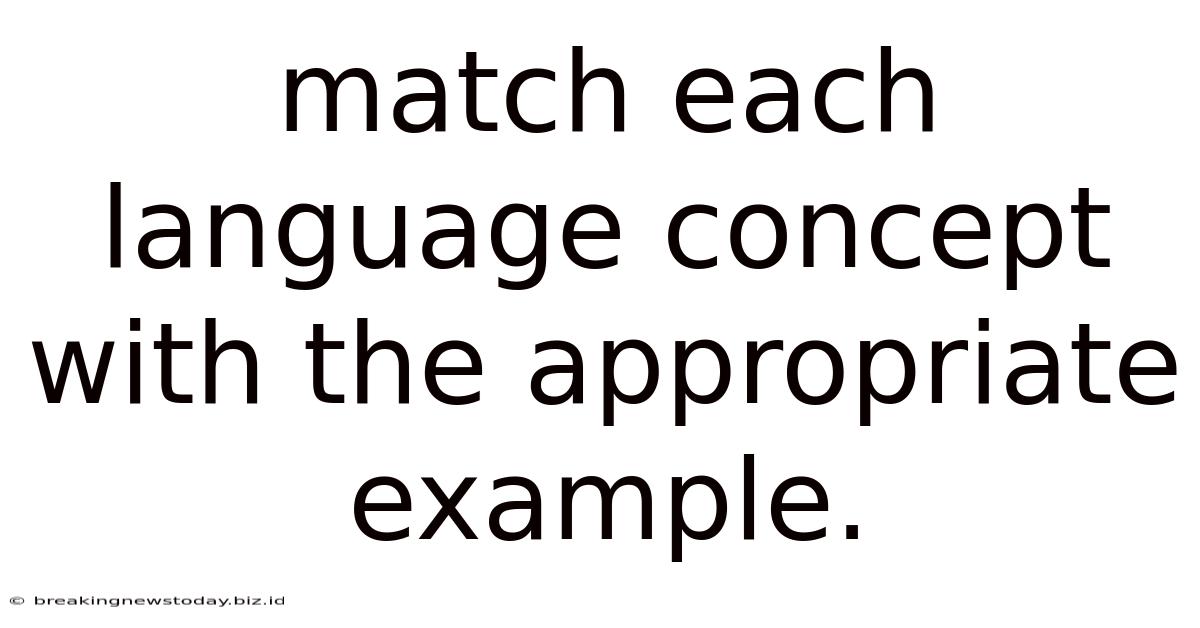
Table of Contents
Match Each Language Concept with the Appropriate Example: A Deep Dive into Programming Fundamentals
Understanding core language concepts is crucial for any aspiring programmer. This comprehensive guide will delve into key concepts, providing clear explanations and illustrative examples in Python, JavaScript, and C++. We'll match each concept with a practical example to solidify your understanding. Let's embark on this journey of clarifying fundamental programming concepts.
1. Variables and Data Types
Variables are symbolic names that represent memory locations storing data. Data types define the kind of data a variable can hold.
1.1. Integer (int)
- Concept: Represents whole numbers without decimal points.
- Examples:
- Python:
age = 30
- JavaScript:
quantity = 100
- C++:
int score = 95;
- Python:
1.2. Floating-Point Number (float)
- Concept: Represents numbers with decimal points.
- Examples:
- Python:
price = 99.99
- JavaScript:
temperature = 25.5
- C++:
float pi = 3.14159;
- Python:
1.3. String (str)
- Concept: Represents sequences of characters.
- Examples:
- Python:
name = "Alice"
- JavaScript:
message = "Hello, world!"
- C++:
std::string greeting = "Good morning";
- Python:
1.4. Boolean (bool)
- Concept: Represents truth values:
True
orFalse
. - Examples:
- Python:
is_active = True
- JavaScript:
isLoggedIn = false
- C++:
bool isValid = true;
- Python:
2. Operators
Operators perform actions on operands (variables or values).
2.1. Arithmetic Operators
- Concept: Perform mathematical calculations. Include +, -, *, /, %, // (floor division), ** (exponentiation).
- Examples:
- Python:
result = 10 + 5 * 2 # Order of operations matters
- JavaScript:
total = (15 / 3) - 2
- C++:
int remainder = 17 % 5;
- Python:
2.2. Comparison Operators
- Concept: Compare two values, resulting in a Boolean value. Include == (equals), != (not equals), > (greater than), < (less than), >= (greater than or equals), <= (less than or equals).
- Examples:
- Python:
is_equal = (x == y)
- JavaScript:
isGreater = (a > b)
- C++:
bool isEqual = (num1 != num2);
- Python:
2.3. Logical Operators
- Concept: Combine Boolean expressions. Include
and
,or
,not
. - Examples:
- Python:
is_valid = (age > 18 and has_license)
- JavaScript:
can_proceed = (is_logged_in || has_permission)
- C++:
bool is_allowed = (temperature > 0 && pressure < 100);
- Python:
3. Control Flow Statements
Control flow statements alter the execution sequence of a program.
3.1. Conditional Statements (if, else if, else)
- Concept: Execute different blocks of code based on conditions.
- Examples:
- Python:
if age >= 18: print("Adult") elif age >= 13: print("Teenager") else: print("Child")
- JavaScript:
if (score > 90) { console.log("A Grade"); } else if (score > 80) { console.log("B Grade"); } else { console.log("Below B Grade"); }
- C++:
if (speed > 100) { std::cout << "Speeding!" << std::endl; } else { std::cout << "Safe speed." << std::endl; }
3.2. Loops (for, while)
- Concept: Repeatedly execute a block of code.
- Examples:
- Python:
# For loop for i in range(5): print(i) # While loop count = 0 while count < 5: print(count) count += 1
- JavaScript:
// For loop for (let i = 0; i < 5; i++) { console.log(i); } // While loop let j = 0; while (j < 5) { console.log(j); j++; }
- C++:
// For loop for (int i = 0; i < 5; i++) { std::cout << i << std::endl; } // While loop int k = 0; while (k < 5) { std::cout << k << std::endl; k++; }
4. Functions
Functions are reusable blocks of code that perform specific tasks.
4.1. Function Definition and Call
- Concept: Defines a function and then calls it to execute the code within.
- Examples:
- Python:
def greet(name): print(f"Hello, {name}!") greet("Bob")
- JavaScript:
function add(x, y) { return x + y; } let sum = add(5, 3); console.log(sum);
- C++:
int multiply(int a, int b) { return a * b; } int product = multiply(4, 6); std::cout << product << std::endl;
4.2. Function Parameters and Return Values
- Concept: Functions can accept parameters (input values) and return values (output values).
- Examples: (The examples above already demonstrate this concept)
5. Arrays and Data Structures
Arrays and other data structures organize and manage data efficiently.
5.1. Arrays (Lists in Python)
- Concept: Ordered collections of elements of the same data type.
- Examples:
- Python:
numbers = [1, 2, 3, 4, 5]
- JavaScript:
colors = ["red", "green", "blue"];
- C++:
int numbers[5] = {1, 2, 3, 4, 5};
- Python:
5.2. Dictionaries (Objects in JavaScript, Maps in C++)
- Concept: Collections of key-value pairs.
- Examples:
- Python:
person = {"name": "Alice", "age": 30}
- JavaScript:
user = { name: "Bob", age: 25 };
- C++:
std::map<std::string, int> ages; ages["Alice"] = 30;
- Python:
6. Object-Oriented Programming (OOP) Concepts
OOP is a programming paradigm that organizes code around "objects" that contain data (attributes) and methods (functions) that operate on that data. While the depth of OOP is extensive, we'll touch upon the basics here.
6.1. Classes and Objects
- Concept: A class is a blueprint for creating objects. An object is an instance of a class.
- Examples:
- Python:
class Dog: def __init__(self, name, breed): self.name = name self.breed = breed def bark(self): print("Woof!") my_dog = Dog("Buddy", "Golden Retriever") my_dog.bark()
- JavaScript:
class Cat { constructor(name, color) { this.name = name; this.color = color; } meow() { console.log("Meow!"); } } let myCat = new Cat("Whiskers", "Gray"); myCat.meow();
- C++:
class Car { public: std::string model; int year; void drive() { std::cout << "Driving the car." << std::endl; } }; int main() { Car myCar; myCar.model = "Toyota Camry"; myCar.year = 2023; myCar.drive(); return 0; }
6.2. Inheritance
- Concept: Creating new classes (child classes) based on existing classes (parent classes), inheriting attributes and methods.
- Examples: (Illustrative, requires a deeper understanding of inheritance to fully implement) This concept is demonstrated in more advanced scenarios where a child class extends the functionality of a parent class. For example, a "GoldenRetriever" class could inherit from a "Dog" class, adding breed-specific characteristics.
6.3. Polymorphism
- Concept: The ability of objects of different classes to respond to the same method call in their own specific way.
- Examples: (Illustrative, requires a deeper understanding of polymorphism) This concept is demonstrated in scenarios where different classes implement a common interface or method signature in their own unique way. For example, different animal classes (Dog, Cat, Bird) could all have a "makeSound()" method, but the implementation of that method would be different for each animal.
7. Exception Handling
Exception handling allows programs to gracefully handle errors.
7.1. Try-Except Blocks (Python)
- Concept: Attempts to execute code within a
try
block and catches potential exceptions in anexcept
block. - Example:
try: result = 10 / 0 except ZeroDivisionError: print("Error: Cannot divide by zero.")
7.2. Try-Catch Blocks (JavaScript)
- Concept: Similar to Python's try-except, attempting code within
try
and catching errors withincatch
. - Example:
try { let result = 10 / 0; } catch (error) { console.error("Error:", error.message); }
7.3. Try-Catch Blocks (C++)
- Concept: Uses
try
,catch
, and optionallythrow
for exception handling. - Example:
#include
#include int main() { try { // some code that might throw an exception throw std::runtime_error("Something went wrong!"); } catch (const std::runtime_error& error) { std::cerr << "Error: " << error.what() << std::endl; } return 0; }
This comprehensive guide provides a solid foundation in fundamental programming language concepts. Remember, consistent practice and exploration are key to mastering these concepts and building your programming skills. Each example provided offers a starting point for further exploration and experimentation. As you progress, consider exploring more advanced topics within each language to deepen your expertise. Remember to consult language-specific documentation for detailed information and further examples.
Latest Posts
Related Post
Thank you for visiting our website which covers about Match Each Language Concept With The Appropriate Example. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.