Most Programmers Use A For Loop _______________.
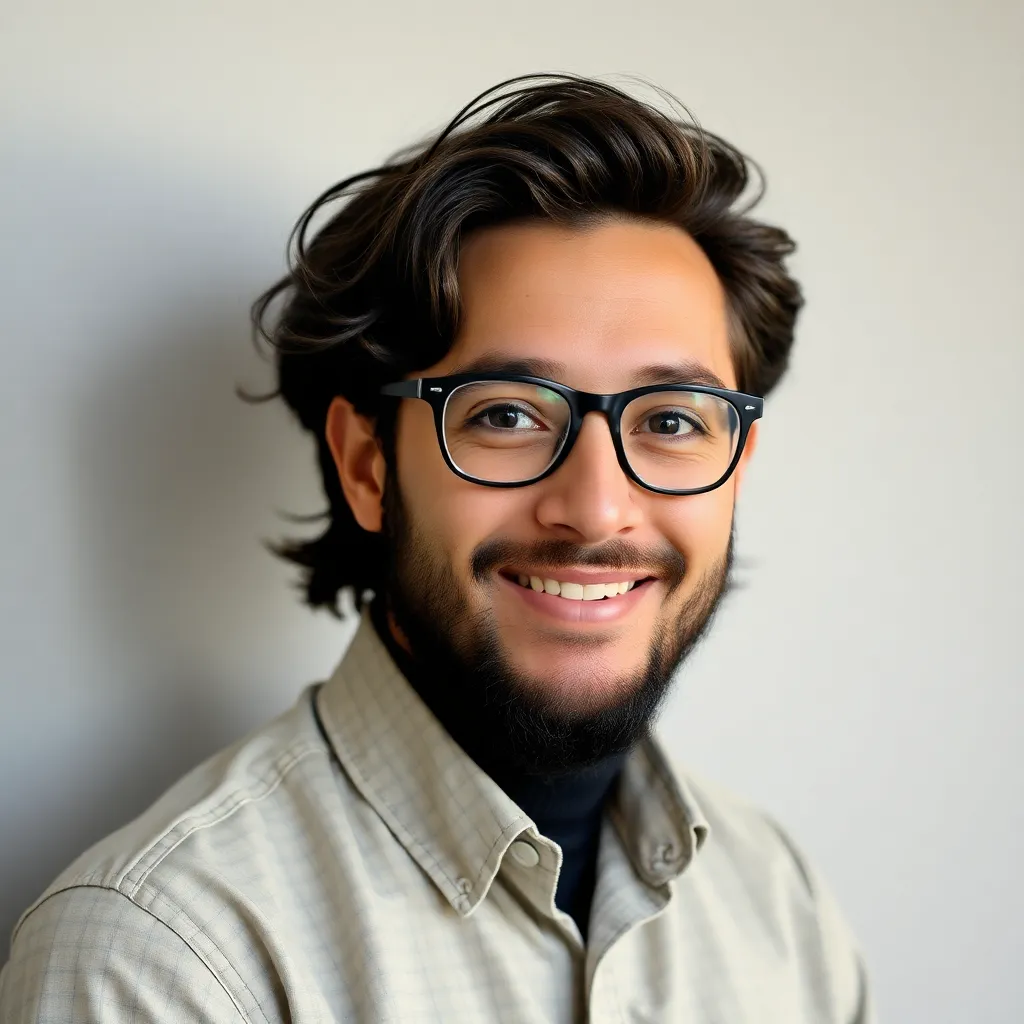
Breaking News Today
Mar 21, 2025 · 6 min read
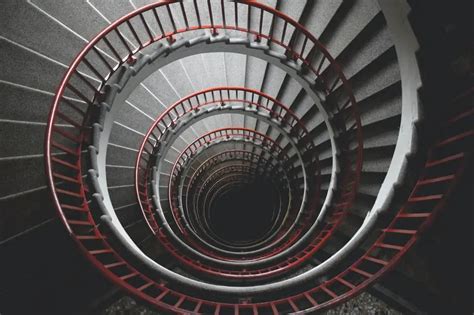
Table of Contents
Most Programmers Use a For Loop…Inefficiently
The humble for
loop. A cornerstone of programming, a workhorse of iteration, a…potential bottleneck? While the for
loop is a fundamental tool in every programmer's arsenal, many developers unknowingly utilize it in ways that significantly impact performance and code readability. This article delves into the common inefficiencies surrounding for
loop usage and presents optimized alternatives to boost your code's speed and elegance.
The Ubiquitous For Loop: A Quick Recap
Before we dissect the inefficiencies, let's establish a common understanding. A for
loop, in its simplest form, iterates over a sequence (like an array or list) or executes a block of code a specified number of times. Its syntax varies slightly across programming languages, but the core concept remains the same. Here's a generic example:
for (initialization; condition; increment) {
// Code to be executed in each iteration
}
This seemingly simple construct can lead to significant performance issues if not used judiciously.
Common Inefficiencies and Their Solutions
Now, let's explore some frequent pitfalls programmers fall into when using for
loops:
1. Unnecessary Array Traversal and Repetitive Calculations
Imagine you need to find the sum of squares of numbers in an array. A naive approach might look like this:
let numbers = [1, 2, 3, 4, 5];
let sumOfSquares = 0;
for (let i = 0; i < numbers.length; i++) {
let square = numbers[i] * numbers[i];
sumOfSquares += square;
}
This code repeatedly accesses the numbers.length
property within the loop condition. While seemingly insignificant for small arrays, this becomes a performance drag for larger datasets. The numbers.length
property is accessed in every single iteration, unnecessarily.
Optimized Solution:
Store the array's length in a variable before the loop:
let numbers = [1, 2, 3, 4, 5];
let sumOfSquares = 0;
let len = numbers.length; // Store length outside the loop
for (let i = 0; i < len; i++) {
sumOfSquares += numbers[i] * numbers[i];
}
This simple change avoids redundant calculations, improving efficiency, especially for large arrays.
2. Improper Use of break
and continue
The break
and continue
statements offer control flow within loops, but their misuse can lead to confusing and inefficient code. Overusing break
can make code harder to follow, while haphazard use of continue
can obscure the logic.
Example of Inefficient Use:
for (int i = 0; i < 1000; i++) {
if (i % 2 == 0) {
continue; // Skip even numbers.
}
// Process odd numbers...
if (i == 500) break; // Exit at 500
}
This code mixes logic for odd number processing with the break
condition. It's less readable and harder to debug than a more structured approach.
Optimized Solution:
Use a more explicit looping structure that avoids unnecessary continue
statements. For instance, you could iterate only through odd numbers:
for (int i = 1; i < 1000; i += 2) {
// Process odd numbers...
if (i == 499) break; // Clearer break condition
}
This revised code is far cleaner, more efficient, and easier to understand. The condition is straightforward, improving readability and maintainability.
3. Inefficient Array Manipulation Inside Loops
Modifying arrays while iterating through them is a common source of errors and performance bottlenecks. The act of adding or removing elements during iteration can shift indices, leading to unexpected behavior.
Example:
my_list = [1, 2, 3, 4, 5]
for i in range(len(my_list)):
if my_list[i] % 2 == 0:
my_list.remove(my_list[i]) # Danger! Modifies during iteration.
This code will likely cause unexpected behavior because removing elements modifies the list's length and indices during iteration, potentially skipping elements.
Optimized Solution:
Create a new list to store the modified results:
my_list = [1, 2, 3, 4, 5]
new_list = []
for item in my_list:
if item % 2 != 0:
new_list.append(item)
my_list = new_list # Assign the new list back to my_list.
This approach avoids modifying the list during iteration, preventing unexpected errors and improving code clarity. Using list comprehensions (Python) or similar functional approaches is an even more concise and efficient alternative:
my_list = [1, 2, 3, 4, 5]
my_list = [item for item in my_list if item % 2 != 0]
This concise form is generally more efficient and easier to read than explicit loops for simple array manipulations.
4. Nested For Loops and Their Complexity
Nested for
loops are often necessary, but they can quickly lead to O(n²) or worse time complexity. This means the execution time grows quadratically with the input size, dramatically impacting performance for large datasets.
Example:
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
// Perform some operation...
}
}
Optimization Strategies:
- Algorithmic Optimization: Analyze the algorithm. Can the problem be solved with a more efficient algorithm that avoids nested loops? Often, a well-chosen data structure or algorithm can significantly reduce time complexity.
- Memoization or Dynamic Programming: If the nested loop involves repeated calculations with the same inputs, memoization (caching results) or dynamic programming can drastically improve performance.
- Parallelization: For computationally intensive nested loops, consider parallelization to distribute the workload across multiple cores, significantly reducing overall execution time.
5. Ignoring Specialized Data Structures
Standard for
loops can be inefficient when dealing with specific data structures. For instance, using a for
loop to search an unsorted array has O(n) complexity. However, using a hash table (or dictionary in Python) for searching allows for O(1) average-case complexity.
Example:
Let's say you need to frequently check if a value exists in a collection.
Inefficient Approach (Using a for
loop with an array):
const myArray = [10, 20, 30, 40, 50];
function checkIfExists(value) {
for (let i = 0; i < myArray.length; i++) {
if (myArray[i] === value) {
return true;
}
}
return false;
}
Efficient Approach (Using a Set):
const mySet = new Set([10, 20, 30, 40, 50]);
function checkIfExists(value) {
return mySet.has(value); // O(1) average-case complexity
}
Using a Set
provides significantly faster lookups than iterating through an array with a for
loop.
Beyond For Loops: Exploring Alternatives
While for
loops are essential, several alternatives exist that often offer greater efficiency or better readability.
forEach
(JavaScript, other languages): Provides a concise way to iterate over arrays without managing indices explicitly.for...of
(JavaScript): Iterates directly over the values of an iterable object.for...in
(JavaScript): Iterates over the keys of an object.- Iterators and Generators: For complex iteration patterns, iterators and generators provide a more elegant and efficient way to traverse data structures.
- Functional Programming Techniques (map, filter, reduce): These higher-order functions allow for concise and efficient array transformations without explicit loops.
- Streams (Java, other languages): For very large datasets, streams enable lazy evaluation and parallel processing, leading to significant performance gains.
Conclusion: Write Efficient Loops, Write Better Code
The for
loop is a powerful tool, but its misuse can lead to significant performance issues. By understanding common inefficiencies and exploring alternative approaches, you can write cleaner, more maintainable, and significantly faster code. Remember to analyze your algorithms, choose appropriate data structures, and leverage the power of functional programming techniques to avoid unnecessary loop iterations and create truly optimized programs. The goal is not simply to use the least amount of code, but to produce highly performant and easily understood solutions. The key to efficient programming often lies in choosing the right tools and techniques for the task at hand, and sometimes, that means moving beyond the ubiquitous but sometimes inefficient for
loop.
Latest Posts
Latest Posts
-
Answers To The American Red Cross Lifeguard Test
Mar 28, 2025
-
How Did Reza Pahlavi Differ From Ayatollah Khomeini
Mar 28, 2025
-
Natural Concepts Are Mental Groupings Created Naturally Through Our
Mar 28, 2025
-
The Gift Of The Magi Answer Key
Mar 28, 2025
Related Post
Thank you for visiting our website which covers about Most Programmers Use A For Loop _______________. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.