Every Complete C++ Program Must Have A
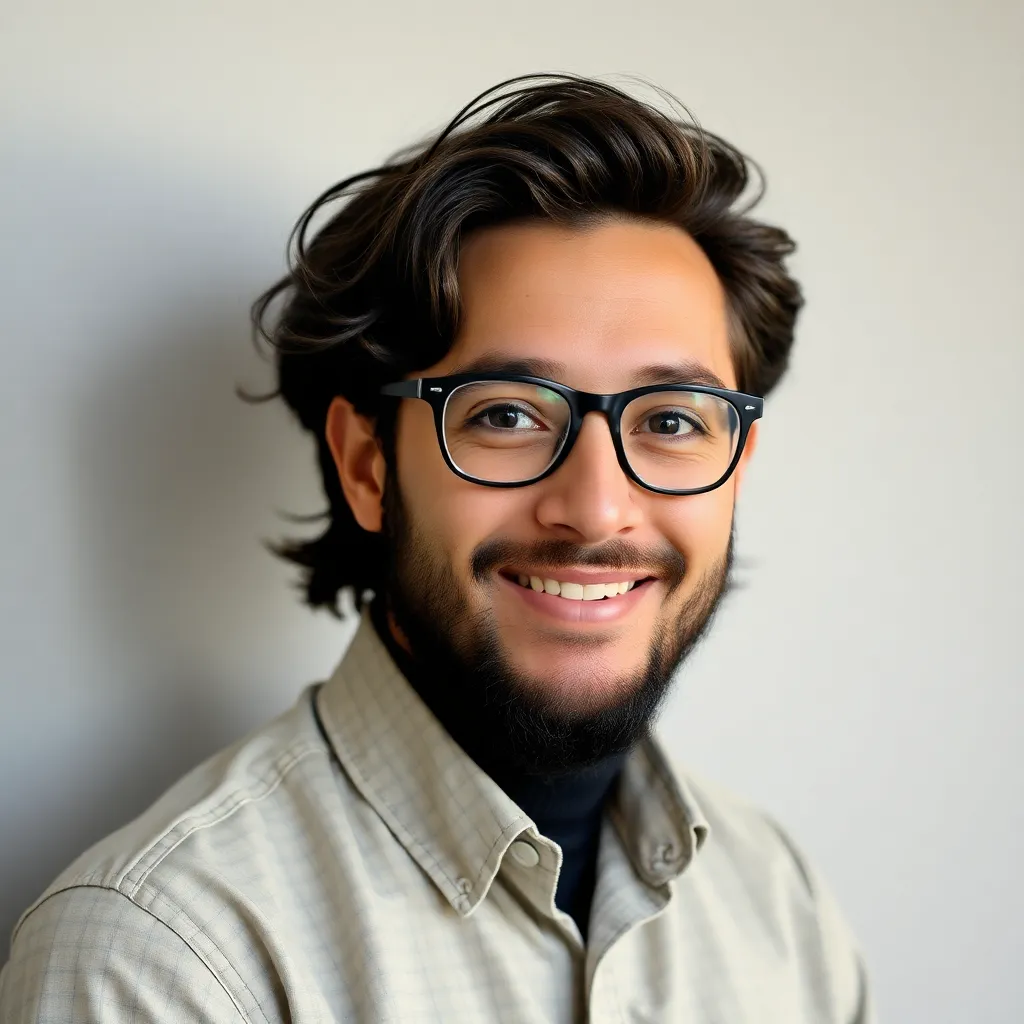
Breaking News Today
Apr 02, 2025 · 5 min read

Table of Contents
Every Complete C++ Program Must Have a main
Function: A Deep Dive
Every C++ program, regardless of its complexity or purpose, needs one fundamental component to function: the main
function. This isn't just a convention; it's the bedrock upon which the entire program is built. Without it, your code won't compile, and it certainly won't run. This article will explore the crucial role of the main
function, its structure, variations, and how it interacts with the rest of your C++ program. We'll delve into best practices and address common misconceptions.
Understanding the main
Function's Role
The main
function serves as the entry point for your C++ program. When you compile and run your code, the operating system's execution begins at this point. Think of it as the ignition switch of your program – it's the first thing that gets executed, and it sets everything in motion. The main
function orchestrates the execution of all other parts of your code, controlling the flow and managing resources.
Key Responsibilities of the main
Function:
- Program Initialization: It often initializes variables, allocates memory, and sets up the necessary environment for the program to run correctly.
- Execution Control: It directs the order in which various functions and code blocks are executed. This is usually done using control structures like
if
,else
,for
, andwhile
statements. - Resource Management: Crucially, it handles the allocation and deallocation of resources, such as memory. Proper resource management is vital to prevent memory leaks and ensure program stability.
- Program Termination: Finally, it signifies the end of the program's execution, often by returning a value to the operating system.
The Structure of the main
Function
The basic structure of a main
function is straightforward:
int main() {
// Your program's code goes here
return 0; // Indicates successful execution
}
Let's break down this structure:
int main()
: This line declares themain
function. Theint
indicates that the function will return an integer value. This returned value is used to signal the operating system about the program's success or failure.{ ... }
: These curly braces enclose the body of themain
function, containing all the code that will be executed.return 0;
: This statement returns the value 0 to the operating system. By convention, 0 signifies successful execution, while non-zero values indicate errors. The specific meaning of non-zero return values is often defined by the program itself or by system conventions.
Variations of the main
Function
While the above structure is the most common, there are a few variations:
int main(int argc, char* argv[])
: This version ofmain
allows you to pass command-line arguments to your program.argc
represents the number of command-line arguments, andargv
is an array of character pointers, each pointing to a command-line argument string. This is highly useful for providing flexibility and user input to your program. For example:
#include
#include
int main(int argc, char* argv[]) {
if (argc > 1) {
std::string argument = argv[1];
std::cout << "The command-line argument is: " << argument << std::endl;
} else {
std::cout << "No command-line argument provided." << std::endl;
}
return 0;
}
int main(int argc, char** argv[])
: This is functionally equivalent to the previous example.
These variations offer greater control and functionality compared to the simpler int main()
.
Return Values from main
The return value from main
is crucial for communication with the operating system. While 0 typically indicates success, other values can signify specific errors. For example, a return value of 1 might indicate a general error, while other values could represent more specific types of failures. Consistent use of return values enhances debugging and error handling.
Best Practices for main
Function Design:
- Keep it Concise: The
main
function should primarily focus on orchestrating the execution of other functions. Avoid placing large blocks of code directly withinmain
. This promotes better code organization and readability. - Modularize your Code: Break down your program's logic into smaller, well-defined functions. This improves maintainability and reduces complexity. The
main
function then acts as a high-level controller, calling these smaller functions as needed. - Error Handling: Implement robust error handling mechanisms. Check for potential errors, such as invalid user input or resource allocation failures. Handle these errors gracefully, providing informative error messages and preventing program crashes.
- Use Meaningful Variable Names: Choose descriptive names for variables and functions to make your code easier to understand.
- Comments: Add comments to explain the purpose and logic of your code. Well-commented code is significantly easier to maintain and debug.
Common Misconceptions about main
main
is just a function: While true, it's a very special function. It's the entry point, and the execution flow begins and ends here.- You can have multiple
main
functions: Absolutely not. A singlemain
function is the only entry point the compiler recognizes. - The return value from
main
is always checked: While it's good practice to check return values from other functions, operating systems don't always explicitly check the return value frommain
. However, returning a non-zero value provides valuable information to the user or a calling process (such as a shell script) about the execution outcome. - You can omit the return statement: Technically, in some compilers, omitting the
return 0;
statement might seem to work. However, it is considered bad practice and can lead to unpredictable behavior. It's always best to explicitly return 0 to indicate successful execution.
Advanced Concepts: main
in Larger Projects
In larger, more complex C++ projects, the main
function may involve more intricate initialization procedures. It may handle the creation of objects, loading of configuration files, and the initialization of other critical components. In these scenarios, the main
function becomes more of a manager than a direct executor of complex tasks. It’s crucial that the design remains modular and easily maintainable.
Conclusion
The main
function is the heart of every C++ program. Understanding its role, structure, variations, and best practices for its implementation is essential for writing well-structured, robust, and maintainable C++ code. While seemingly simple, the main
function provides a crucial foundation upon which the entire program's execution is built. Paying close attention to its design and implementation is a key step towards becoming a proficient C++ programmer. Remember to maintain a clean, well-commented, and modular design, and your C++ programs will be more robust, easier to debug, and simpler to extend in the future. Mastering the main
function is a foundational step in mastering the C++ programming language itself.
Latest Posts
Latest Posts
-
In Meiosis How Does Prophase I Differ From Prophase Ii
Apr 03, 2025
-
Sleep Awareness Week Begins In The Spring With The Release
Apr 03, 2025
-
Based On The Molecular Structures Shown In The Figure
Apr 03, 2025
-
Common Exclusions To Continuation Of Group Coverage Include
Apr 03, 2025
-
Which Of The Following Events Would Increase Producer Surplus
Apr 03, 2025
Related Post
Thank you for visiting our website which covers about Every Complete C++ Program Must Have A . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.